Utilizing Laravel Scout To enable Full-Text Search - (r)
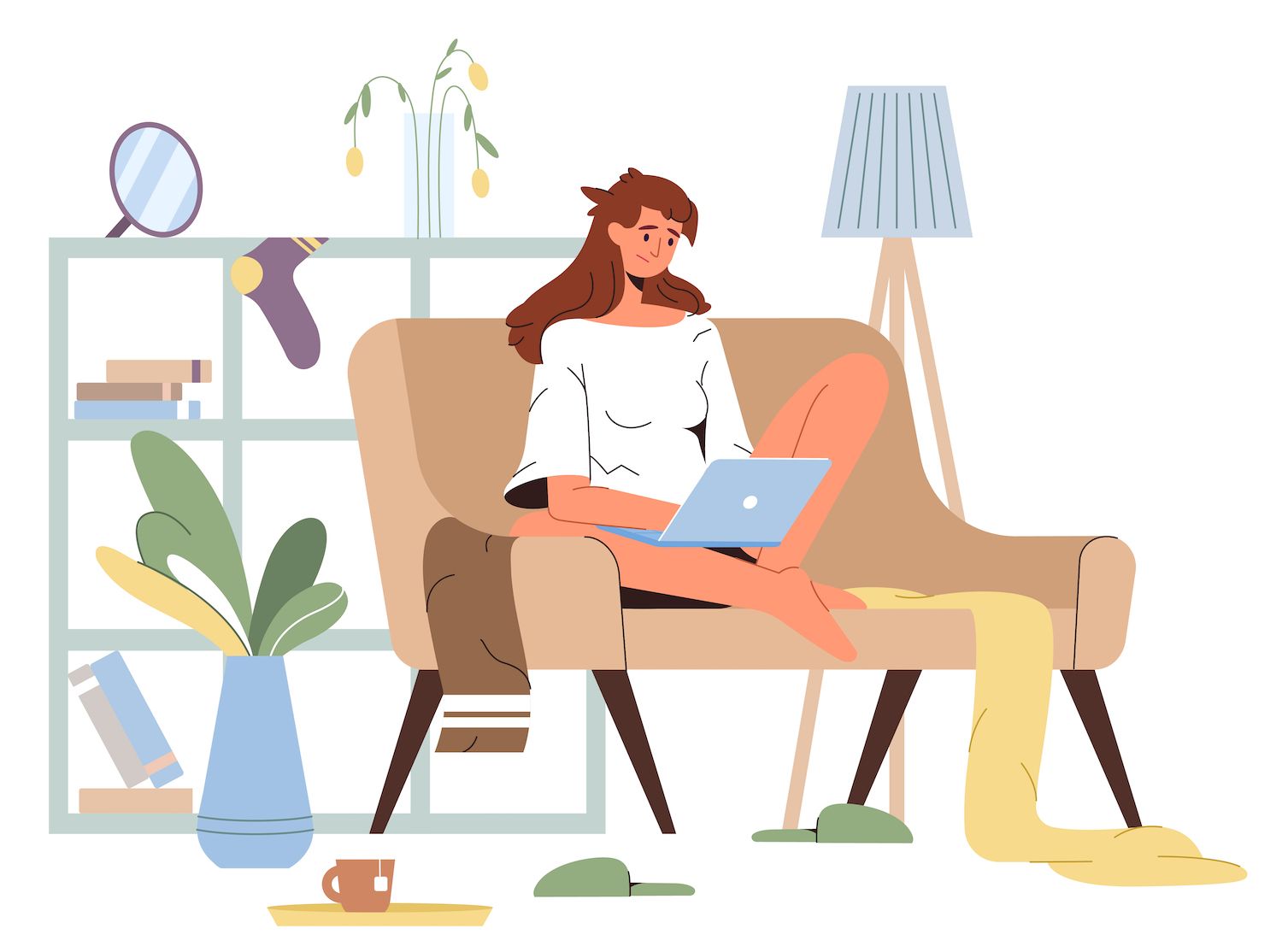
Share the news on
Since it is an open source tool, Laravel offers a myriad of out-of-the-box functionalities that enable creators to design efficient and useful applications.
In this article, we'll explore the tools in depth, and show the user to include the search function in full text to a Laravel application by using the driver. The model will be a Laravel application that stores the names of mockup trains and then make use of Laravel Scout to provide an option to search in the application.
Prerequisites
To follow along with this, you'll need:
- The PHP compiler is installed on your computer. This tutorial uses PHP Version 8.1.
- This is Docker Engine or Docker Desktop you can install on your computer
- A Algolia cloud-based account that you can make for free
How do I install Scout inside a Laravel Project
To be able to use Scout it is necessary to create a Laravel application to include the search feature. The Laravel-Scout Bash script includes the required commands to build an Laravel application that is contained within the Docker container. Docker is a great choice for developers. Docker signifies that there is no have to install any other software including MySQL. MySQL database.
The Laravel-scout script is an application of that Bash scripting language. Therefore, you have to execute it in an Linux environment. If you're using Windows Make sure to configure Windows Subsystem in order to switch it over to Linux (WSL).
If you're running WSL follow the command on your terminal to select your preference for Linux distribution.
WSL -S Ubuntu
Then, go to the section where you'd like to save the project. The Laravel-Scout script will generate the directory for your project at this point. Below is an example of how the Laravel-Scout script would create a directory within the directory. desktop directory.
Cd desktop
Utilize the following command to start the Laravel-Scout program. This will create an Dockerized application by providing boilerplate code needed.
curl -s https://laravel.build/laravel-scout-app | bash
After the process is completed, you can modify your directory with the cd command laravelscout
. Next, execute your sail-up
command from within the directory of your project to launch with the Docker containers running the app.
Note: On many Linux distributions, it is possible to execute the following commands with the sudo
command to be granted elevated rights.
./vendor/bin/sail up
You might encounter an error:
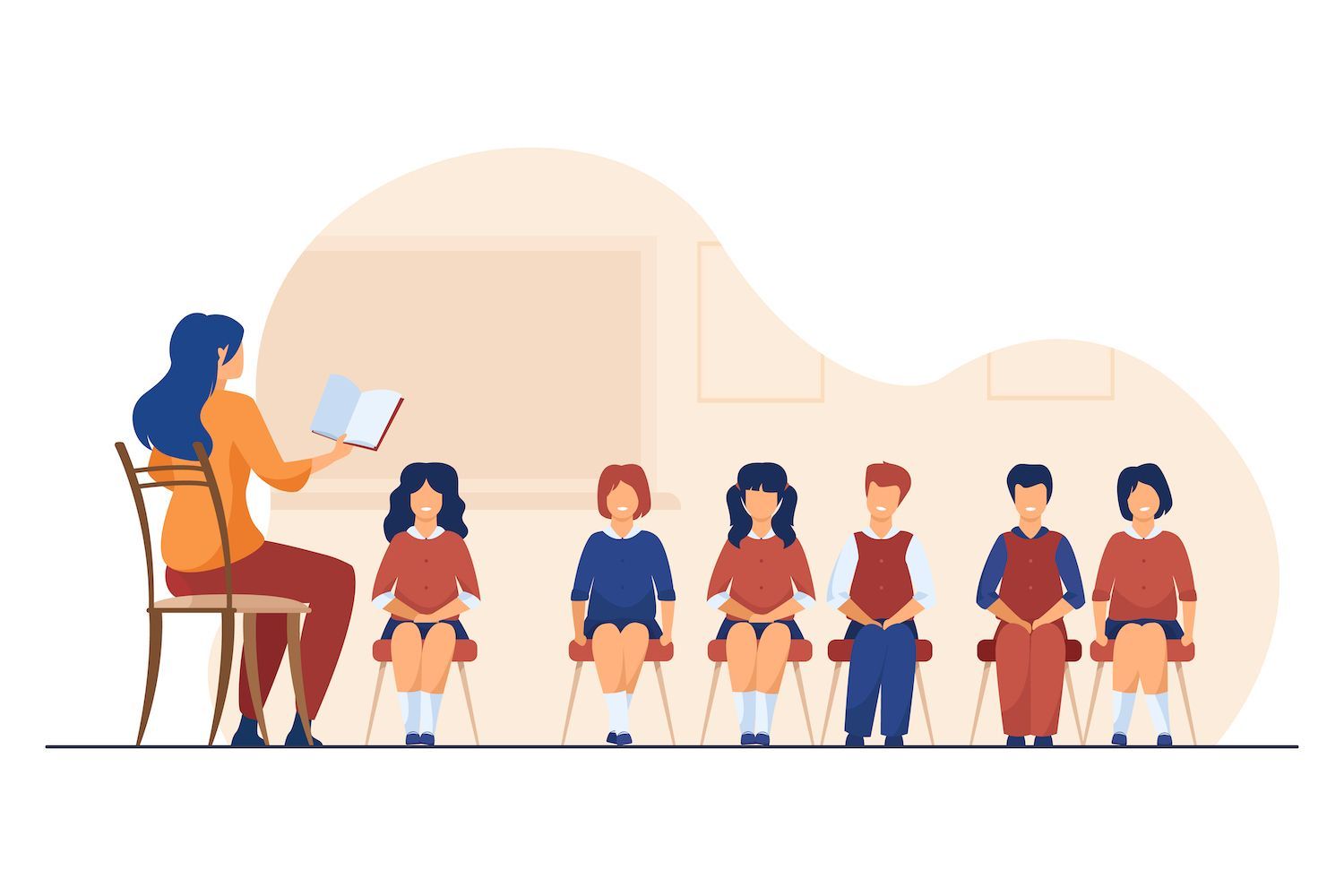
For resolving this issue, utilize the app_port
variable to define the port in sail up: sailing up
command:
APP_PORT=3001 ./vendor/bin/sail up
Then, run the command below to begin the application using Artisan in the PHP. The PHP server.
php artisan serve
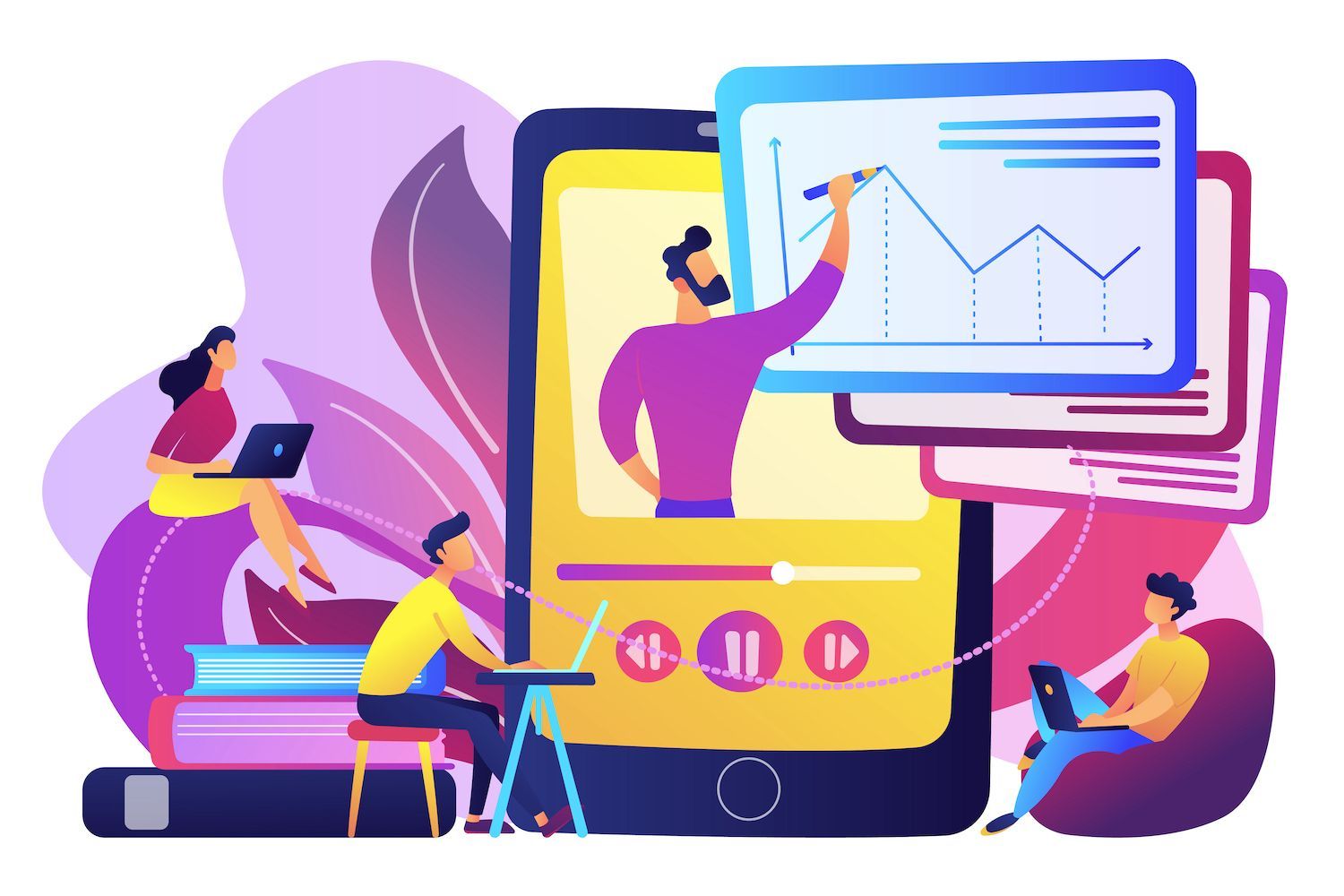
From your web browser, navigate to the running application at http://127.0.0.1:8000. The program will open your Laravel welcome page at the URL you are used to.
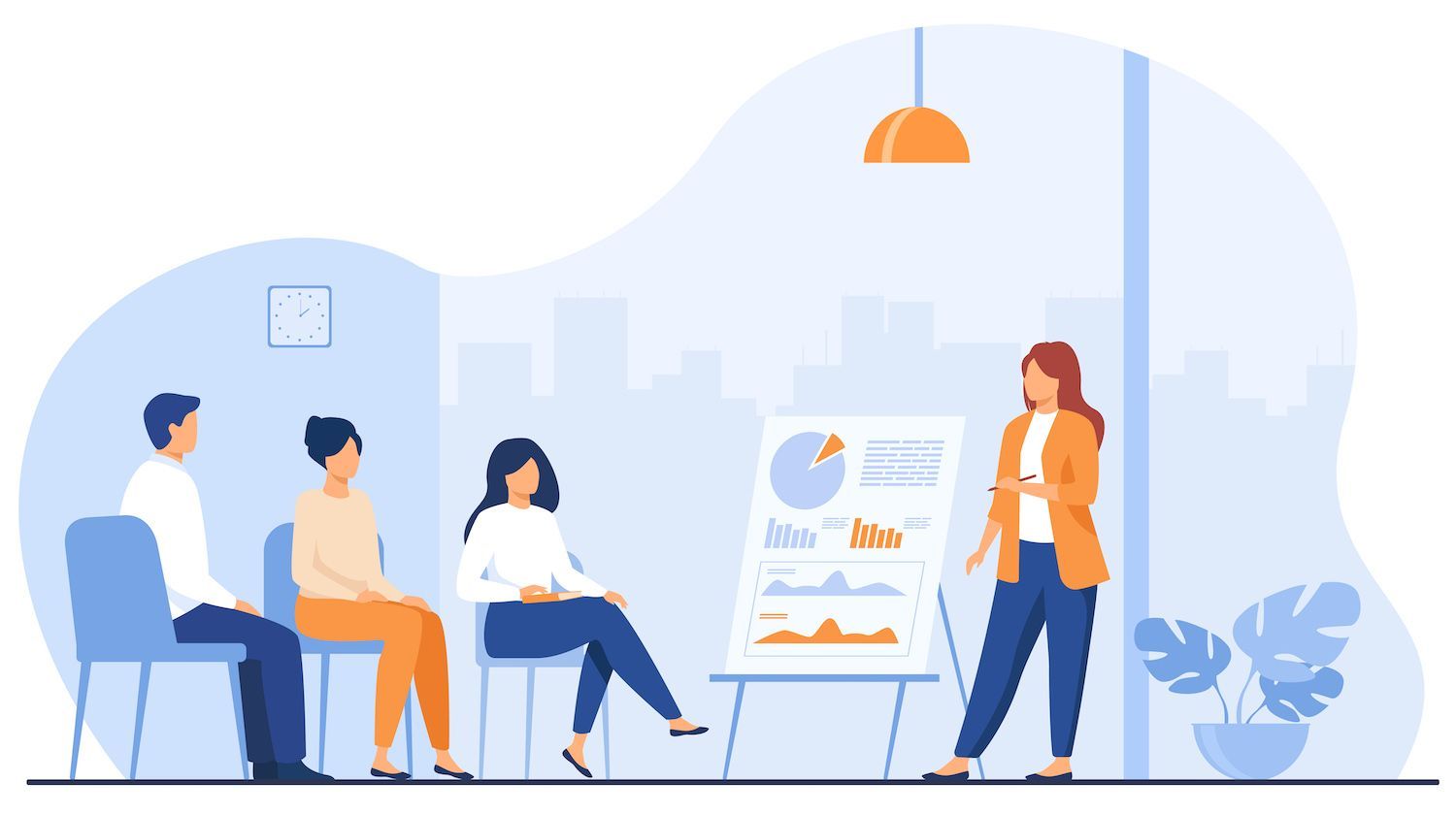
How do I add Laravel Scout into the App
In your terminal, type this command to allow your Composer PHP module manager. This will allow Laravel Scout to the project.
composer require laravel/scout
Then, you can publish your Scout configuration file using this command provided by the vendor. The command will publish your scout.php
configuration file to your program's settings directory.
php artisan vendor:publish --provider="Laravel\Scout\ScoutServiceProvider"
Change the boilerplate .env file to include the SCOUT_QUEUE
variable, which is a boolean.
SCOUT_QUEUE SCOUT_QUEUE
value allows Scout to hold operations in queue, which results in faster responses. If it is not set, Scout drivers like Meilisearch won't be able to reflect newly added data promptly.
SCOUT_QUEUE=true
DB_HOST=127.0.0.1
How to Mark a Model and Configure the Index
Scout doesn't enable searchable models of data by default. You must explicitly mark a model as searchable using its Laravel\Scout\Searchable
trait.
You'll start by creating the model for data in an example Train
application and marking the model as searchable.
How do you create a Model
To use the train
application, it is essential to save the names that are placeholders for every train that is available.
Make use of to use the Artisan command to create the migration. Give it the name the table create_trains.
.
php artisan make:migration create_trains_table
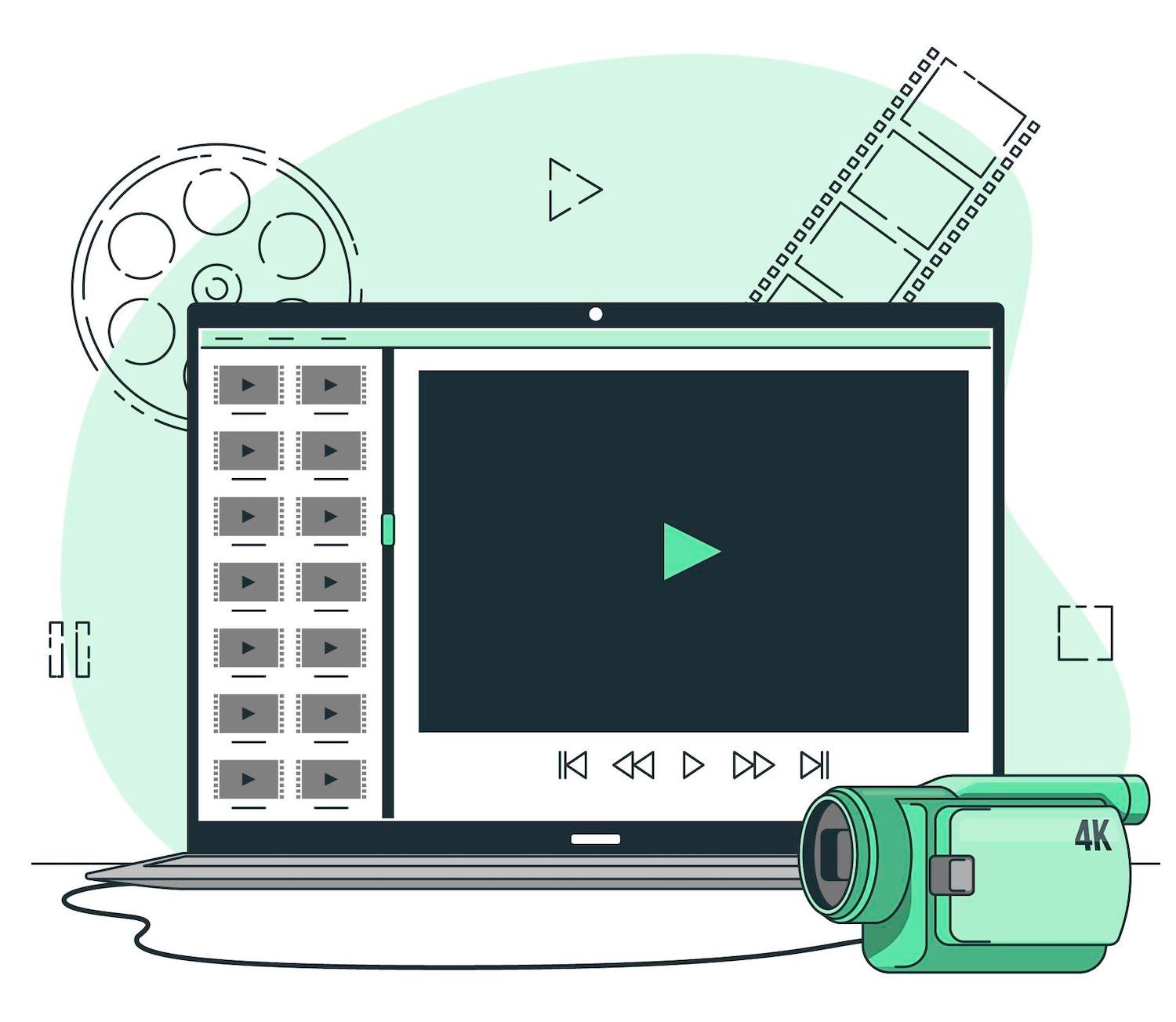
The information is stored within a file that will be a mixture of your name and the time stamp of the time.
Navigate to the migration file in the database/migrationsdirectory. directory.
In order to include an additional title column copy and paste this code following that () column in line 17.()
column in line 17. This code adds the title column.
$table->string('title');
To make the change follow the steps below.
php artisan migrate
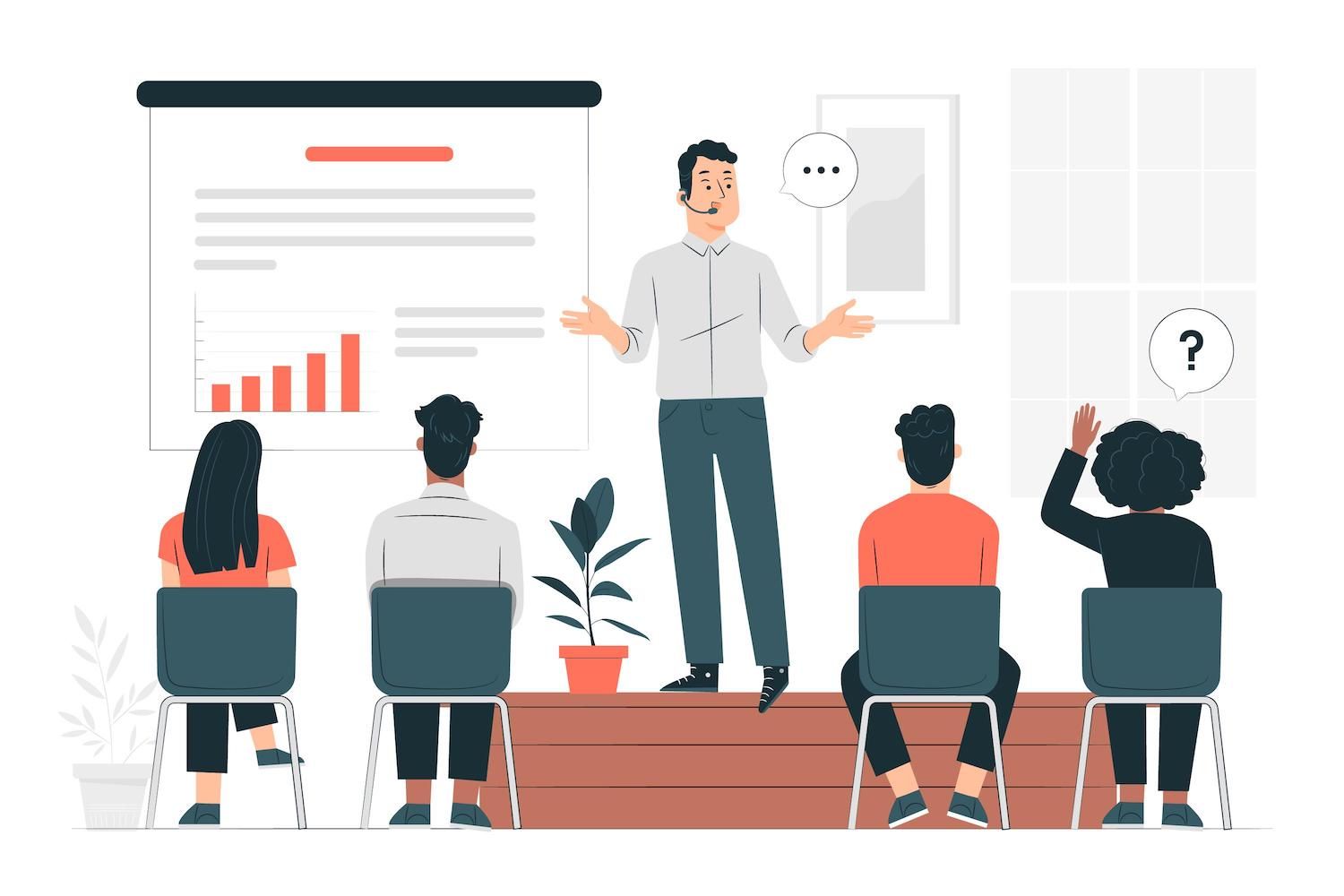
When you have completed the database migrations and creating a file titled Train.php in the model/app directory.
How To Add the LaravelScoutSearchable Trait
Mark the Train
model for search by adding the Laravel\Scout\Searchable
trait to the model, as shown below.
How to use Algolia with Scout
For your first full-text search that you could do using Laravel Scout You'll need the Algolia driver. Algolia is a software as a services (SaaS) system that permits you to browse through vast quantities of data. The platform provides a dashboard on the web for developers who manage their search engines as in addition to an API offering robust interfaces that users are able to access via using the Software Development Kit (SDK) with the programming language of your choice.
Within the Laravel application, you'll utilize it with it with the Algolia client program that is designed for PHP.
How do I set up Algolia
To begin, you need to download the Algolia search client PHP application for your application.
Please follow the directions below.
composer require algolia/algoliasearch-client-php
Next, you must set the Application ID and Secret API Key credentials from Algolia in the .env file.
Utilizing your web browser browse through your Algolia dashboard for the Application ID and secret API Key credentials.
Click Settings at the bottom of the left-hand sidebar. You will be taken to Settings. settings page.
Then, select to view API Keys under The access and team section on the Settings page. You will be able to see the API keys for the account you have created. Algolia account.
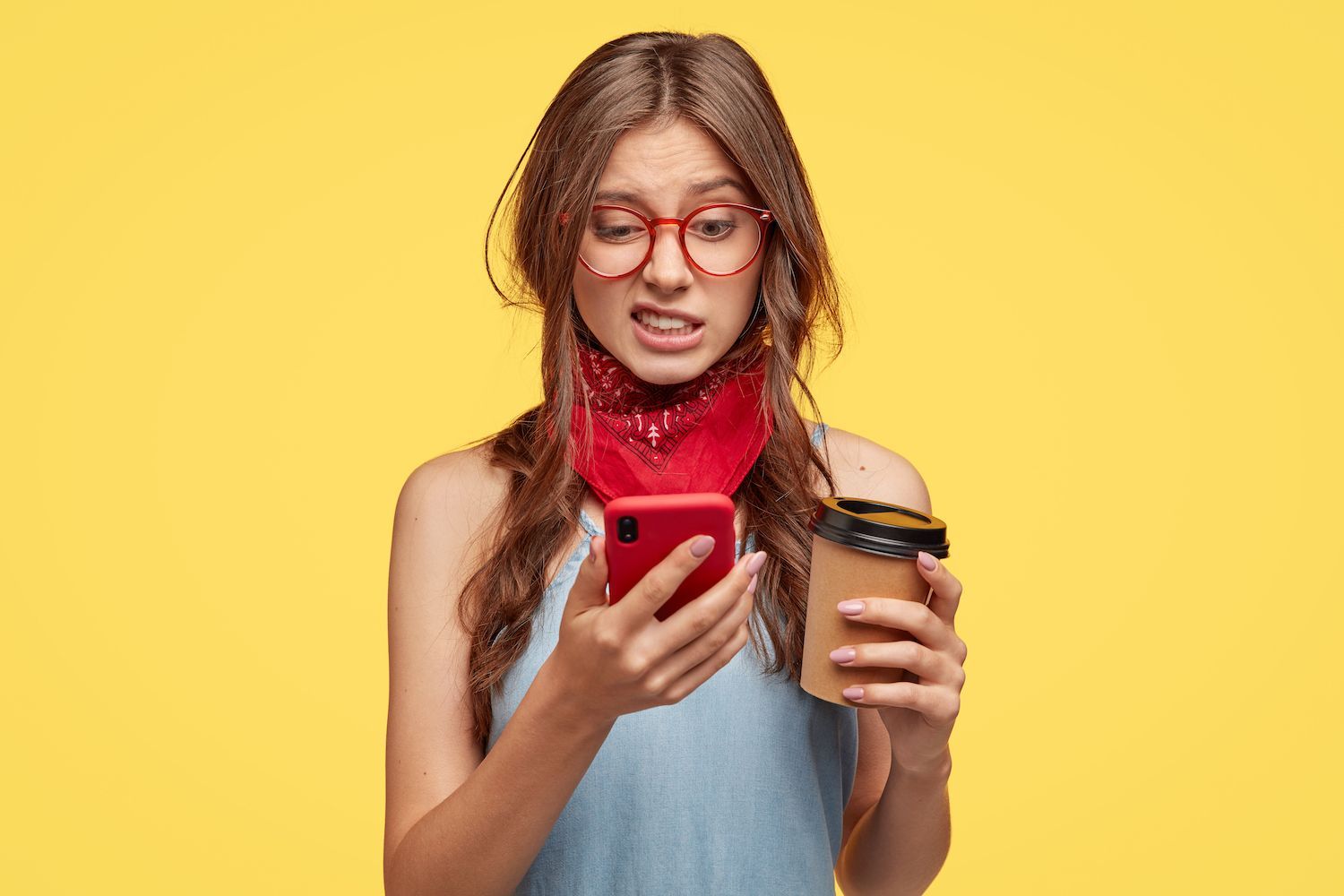
When you visit the API Keys page, take note of the App ID as well as the the Admin API Key value. The credentials are used to verify an exchange of data between Laravel application and Algolia.
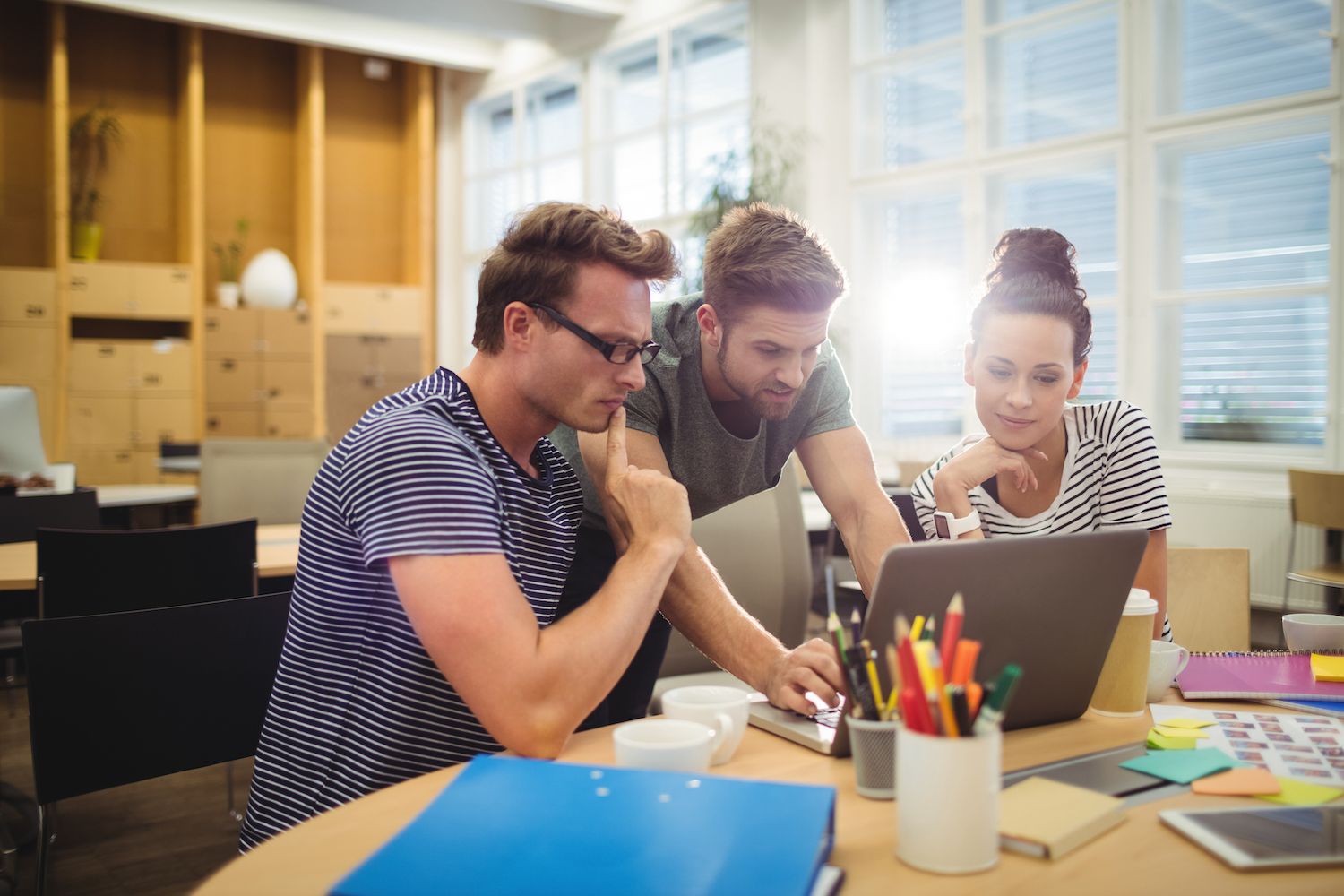
Integrate the following code into your .env file by using your code editor and replace those placeholders by using the relevant Algolia API secrets.
ALGOLIA_APP_ID=APPLICATION_ID ALGOLIA_SECRET=ADMIN_API_KEY
In addition, you could replace also the SCOUT_DRIVER
variable by using this program to change the value from meilisearch
to algolia
. This will instruct Scout to utilize algolia instead. Algolia driver.
SCOUT_DRIVER=algolia
How do I make the Application Controllers
Within the app/Http/Controllers/ directory, create a TrainSearchController.php file to store a controller for the application. It will display and update data in its Train
model.
Add the following code block into the TrainSearchController.php file to build the controller.
has('titlesearch')) $trains = Train::search($request->titlesearch) ->paginate(6); else $trains = Train::paginate(6); return view('Train-search',compact('trains')); /** * Get the index name for the model. * * @return string */ public function create(Request $request) $this->validate($request,['title'=>'required']); $trains = Train::create($request->all()); return back();
How do I create an Application Routes
In this stage, you'll build the routes that will be listed and add trains to the database.
Browse through the routes/web.php file and replace the existing code by the block below.
name ('trains-lists'); Route::post('create-item', [TrainSearchController::class, 'create']) -> name ('create-item');
The following code generates two routes for the application. The one that is a GET
request from the trains-lists
route shows all stored train information. An POST
request from the "/create"
route will be utilized to generate new train data.
How to Create an Application Views
Create a file within the resources/views/ directory and name it Train-search.blade.php. The file will display the user interface that will allow you to search.
Add the content of the code block below into the Train-search.blade.php file to create a single page for the search functionality.
!DOCTYPE HTML> HTML> Laravel - Laravel Scout Algolia Search Examples "container">Laravel Full-Text search using Scout The Scout Autocomplete="off"@if(count($errors)) Whoops! You've encountered an error as you enter your data. @foreach($errors->all() as $error) $error @endforeach @endif $errors->first('title') Create New Train Train Management Search @if($trains->count()) @foreach($trains as $key => $item) @endforeach @else @endif Id Train Title Creation Date Updated Date ++$key $item->title $item->created_at $item->updated_at No train data available $trains->links()
The HTML code shown above has a form element, which has an input field, as well as an option to input the name of a train, prior to saving it into a database. It also contains an HTML table which displays the ID, title, created_at, as well as updated_at information about the train's database entry.
How do I best to use Algolia Search
To view the page, navigate to http://127.0.0.1:8000/trains-lists from your web browser.
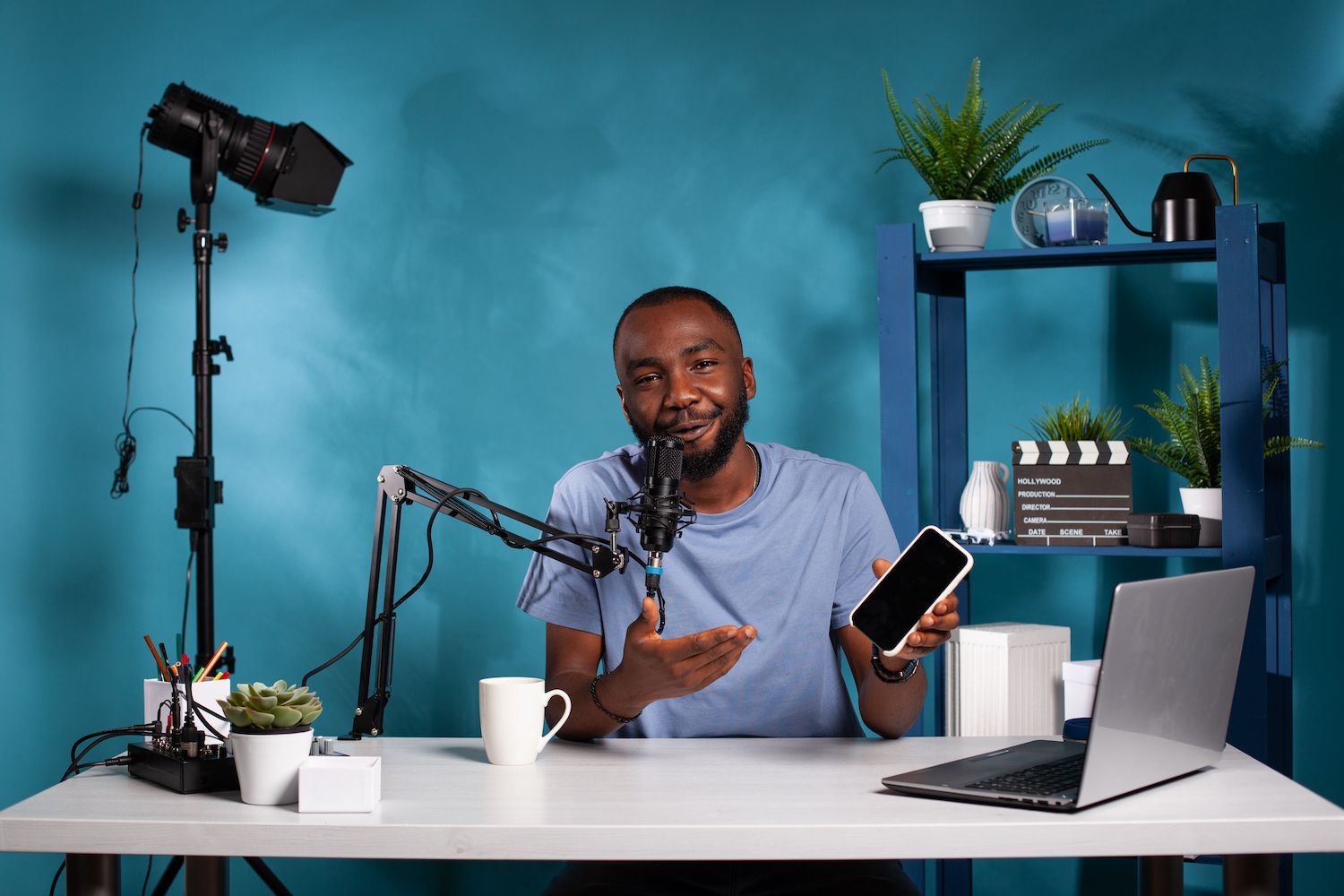
The database isn't yet fully loaded, so you must enter the name of your demo train in the input box, and then click Create New Train to save the train.
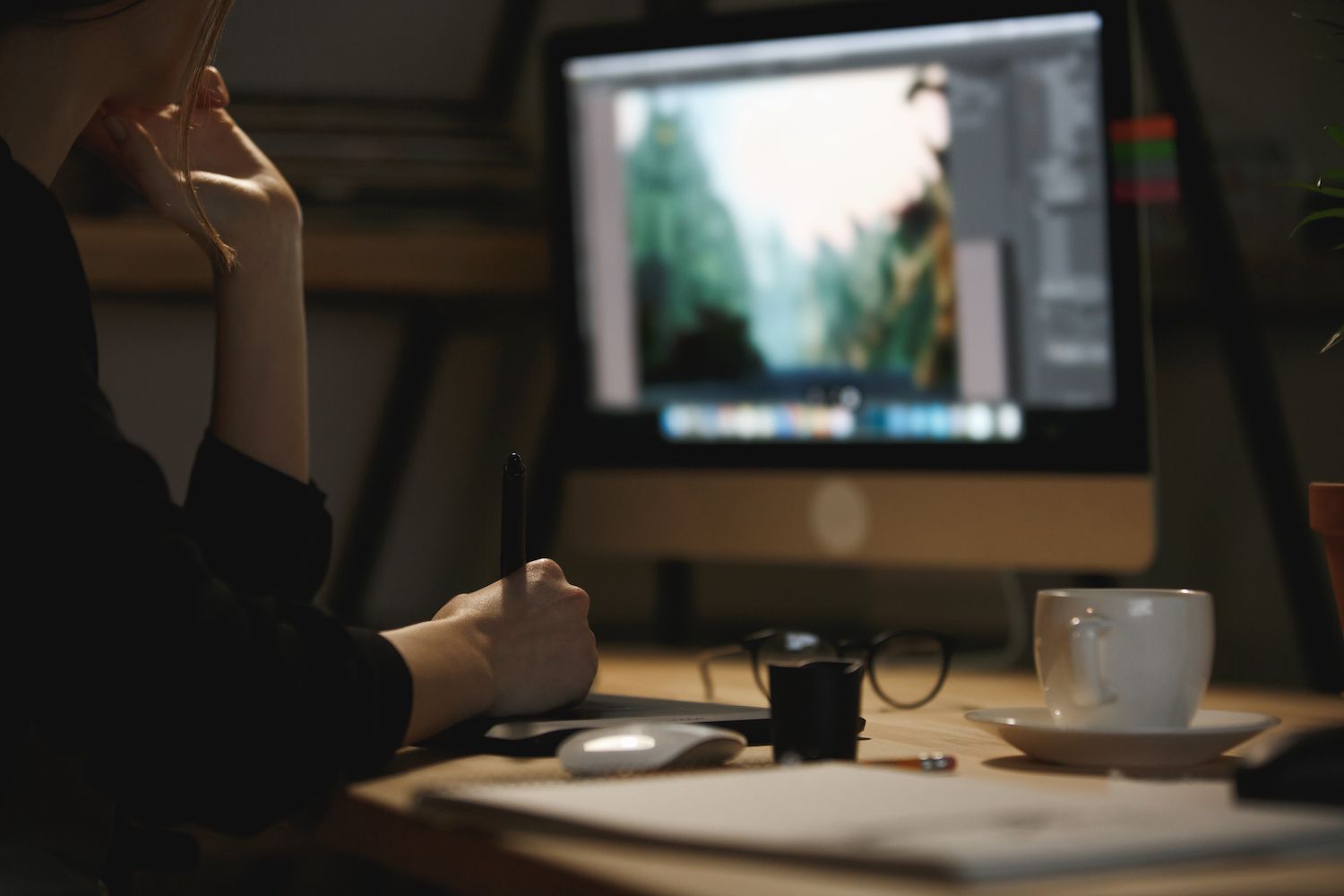
To search for a keyword to search, type in a keyword from any trains you've saved into the Input Title For Search input box and then hit search.
As you can see in the photo below As you can see in the picture below, only entries that include the term "keyword" in their title will display.
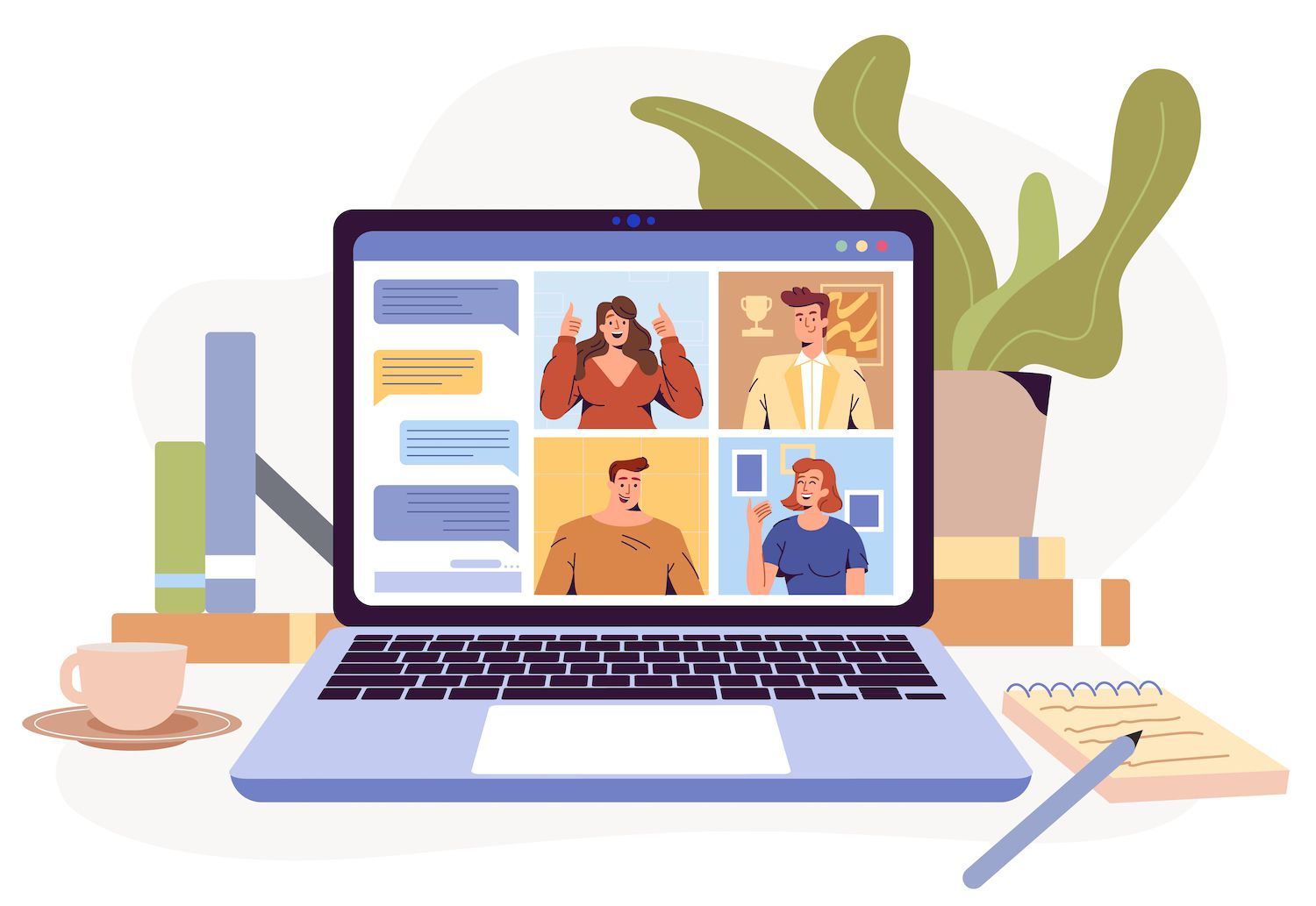
Meilisearch, in conjunction with Laravel Scout
Developers can create and self-host an Meilisearch account on their cloud or in-house infrastructure. Meilisearch also offers a free test cloud offering like Algolia to developers that want to use the product without the need to manage the infrastructure.
In order to integrate Meilisearch with Laravel it is necessary to install Meilisearch. Laravel application, use the following command from the project's terminal.
composer require meilisearch/meilisearch-php
In the next step, you must modify the Meilisearch variables within your .env file to make it more user-friendly.
Replacing the SCOUT_DRIVER
, MEILISEARCH_HOST
and MEILISEARCH_KEY
variables in the .env file with those below.
SCOUT_DRIVER=meilisearch MEILISEARCH_HOST=http://127.0.0.1:7700 MEILISEARCH_KEY=LockKey
The SCOUT_DRIVER
key is the driver Scout is supposed to use. MEILISEARCH_HOST
is the name of the domain in which the Meilisearch instance you have is. While not necessary in the development phase, adding MEILISEARCH_KEY
in production is recommended.
Note: Comment out the Algolia ID and Secret when choosing Meilisearch as your preferred driver.
After you've completed your .env configurations, you need to index your existing entries using the Artisan command as follows.
PHP artisan Scout: import "App\Models\Train"
Laravel Scout is equipped with Database Engine
This engine utilizes "where-like" clauses, along with full-text and indexes of your database to locate the most appropriate outcomes for your search. It is not necessary for indexing your database to be able to utilize the engine in your the database.
For the database engine for it to function, change the SCOUT_DRIVER
.env variable to the database.
Open by opening the .env file within the Laravel application. Change to the values of the SCOUT_DRIVER
variable.
SCOUT_DRIVER = database
When you switch your driver from the database engine, Scout is able to switch to the database engine to perform full-text searches.
Collection Engine with Laravel Scout
In addition to the database engine, Scout provides a collection engine. The engine uses "where" clauses and filters for collections to determine the results that are most relevant to your search.
Contrary to the database engine collection engine can be used in conjunction with the vast majority of relational databases Laravel can also assist with.
You can use the engine to collect by changing the SCOUT_DRIVER
environment variable to the collection
or by manually specifying the collection driver within the Scout Configuration file.
SCOUT_DRIVER is collection
Explorer and Elasticsearch
Thanks to the strengths in Elasticsearch queries, Explorer can be described as a contemporary Elasticsearch driver which works with Laravel Scout. Explorer is a fully compatible Scout driver, and offers benefits such as the ability to save, search and analysing massive quantities of information in real-time. Elasticsearch is integrated with Laravel offers the results within milliseconds.
In order to use Elasticsearch Explorer, the Elasticsearch Explorer driver, in the Laravel program, you'll need to set up your boilerplate docker-compose.yml file that the Laravel-Scout script created. Then, you'll need to add additional settings to Elasticsearch and then restart the containers.
Navigate to your docker-compose.yml file and alter its contents by using the next.
# For more information: https://laravel.com/docs/sail version: '3' services: laravel.test: build: context: ./vendor/laravel/sail/runtimes/8.1 dockerfile: Dockerfile args: WWWGROUP: '$WWWGROUP' image: sail-8.1/app extra_hosts: - 'host.docker.internal:host-gateway' ports: - '$APP_PORT:-80:80' - '$VITE_PORT:-5173:$VITE_PORT:-5173' environment: WWWUSER: '$WWWUSER' LARAVEL_SAIL: 1 XDEBUG_MODE: '$SAIL_XDEBUG_MODE:-off' XDEBUG_CONFIG: '$SAIL_XDEBUG_CONFIG:-client_host=host.docker.internal' volumes: - '. :/var/www/html' networks: - sail depends_on: - mysql - redis - meilisearch - mailhog - selenium - pgsql - elasticsearch mysql: image: 'mysql/mysql-server:8.0' ports: - '$FORWARD_DB_PORT:-3306:3306' environment: MYSQL_ROOT_PASSWORD: '$DB_PASSWORD' MYSQL_ROOT_HOST: "%" MYSQL_DATABASE: '$DB_DATABASE' MYSQL_USER: '$DB_USERNAME' MYSQL_PASSWORD: '$DB_PASSWORD' MYSQL_ALLOW_EMPTY_PASSWORD: 1 volumes: - 'sail-mysql:/var/lib/mysql' - './vendor/laravel/sail/database/mysql/create-testing-database.sh:/docker-entrypoint-initdb.d/10-create-testing-database.sh' networks: - sail healthcheck: test: ["CMD", "mysqladmin", "ping", "-p$DB_PASSWORD"] retries: 3 timeout: 5s elasticsearch: image: 'elasticsearch:7.13.4' environment: - discovery.type=single-node ports: - '9200:9200' - '9300:9300' volumes: - 'sailelasticsearch:/usr/share/elasticsearch/data' networks: - sail kibana: image: 'kibana:7.13.4' environment: - elasticsearch.hosts=http://elasticsearch:9200 ports: - '5601:5601' networks: - sail depends_on: - elasticsearch redis: image: 'redis:alpine' ports: - '$FORWARD_REDIS_PORT:-6379:6379' volumes: - 'sail-redis:/data' networks: - sail healthcheck: test: ["CMD", "redis-cli", "ping"] retries: 3 timeout: 5s pgsql: image: 'postgres:13' ports: - '$FORWARD_DB_PORT:-5432:5432' environment: PGPASSWORD: '$DB_PASSWORD:-secret' POSTGRES_DB: '$DB_DATABASE' POSTGRES_USER: '$DB_USERNAME' POSTGRES_PASSWORD: '$DB_PASSWORD:-secret' volumes: - 'sailpgsql:/var/lib/postgresql/data' networks: - sail healthcheck: test: ["CMD", "pg_isready", "-q", "-d", "$DB_DATABASE", "-U", "$DB_USERNAME"] retries: 3 timeout: 5s meilisearch: image: 'getmeili/meilisearch:latest' ports: - '$FORWARD_MEILISEARCH_PORT:-7700:7700' volumes: - 'sail-meilisearch:/meili_data' networks: - sail healthcheck: test: ["CMD", "wget", "--no-verbose", "--spider", "http://localhost:7700/health"] retries: 3 timeout: 5s mailhog: image: 'mailhog/mailhog:latest' ports: - '$FORWARD_MAILHOG_PORT:-1025:1025' - '$FORWARD_MAILHOG_DASHBOARD_PORT:-8025:8025' networks: - sail selenium: image: 'selenium/standalone-chrome' extra_hosts: - 'host.docker.internal:host-gateway' volumes: - '/dev/shm:/dev/shm' networks: - sail networks: sail: driver: bridge volumes: sail-mysql: driver: local sail-redis: driver: local sail-meilisearch: driver: local sailpgsql: driver: local sailelasticsearch: driver: local
You can then run the command below to get the most current Elasticsearch image which you've added to the docker-compose.yml file.
docker-composes up
Use the Composer feature below to add Explorer within the project.
composer require jeroen-g/explorer
Additionally, you need to make an administrator file to use the Explorer driver.
Use the Artisan command to create the explorer.config file for storage of settings.
php artisan vendor:publish --tag=explorer.config
The configuration file created above can be found in the directory called /config directory.
In the config/explorer.php file, it's possible to refer the model you have created using the indexes
key.
'indexes' => [ \App\Models\Train::class ],
Modify the values of the SCOUT_DRIVER
variable in the .env file to the value of elastic
to allow Scout to run the Explorer driver.
SCOUT_DRIVER = elastic
In this case it's your turn to implement Explorer in your Train
model by using the Explorer interface and then overriding mappableAs()
method.
Start the Train.php file within the App Models directory. Replace your existing code by the following code.
$this->Id, 'title' => $this->title, ];
By using the code you included the above code, you'll be able to access Explorer to search for text within this model. Train
model.
Summary
To PHP designers, Laravel and add-ons like Scout make it a breeze to integrate fast and powerful full-text search functions. By using Laravel's Database Engine, Collection Engine as well as the features offered with Meilisearch and Elasticsearch that allow you to connect with your database's data and incorporate advanced search features within milliseconds.
It is easy to manage your database and keeping it up-to-date ensures that your customers get the best possible experience while your code stays clean and streamlined.
- Simple setup and management on My dashboard. My dashboard
- Support is always available.
- The most powerful Google Cloud Platform hardware and network is powered by Kubernetes to guarantee maximum capacity
- The most sophisticated Cloudflare integration that speeds up and security
- The reach of the global viewers is enhanced by as many as 35 data centers, and more than 275 PoPs across the world
This post was first seen on here