Slow loading on Angular (Put it into Action on Your Web Site) -(r) (r)
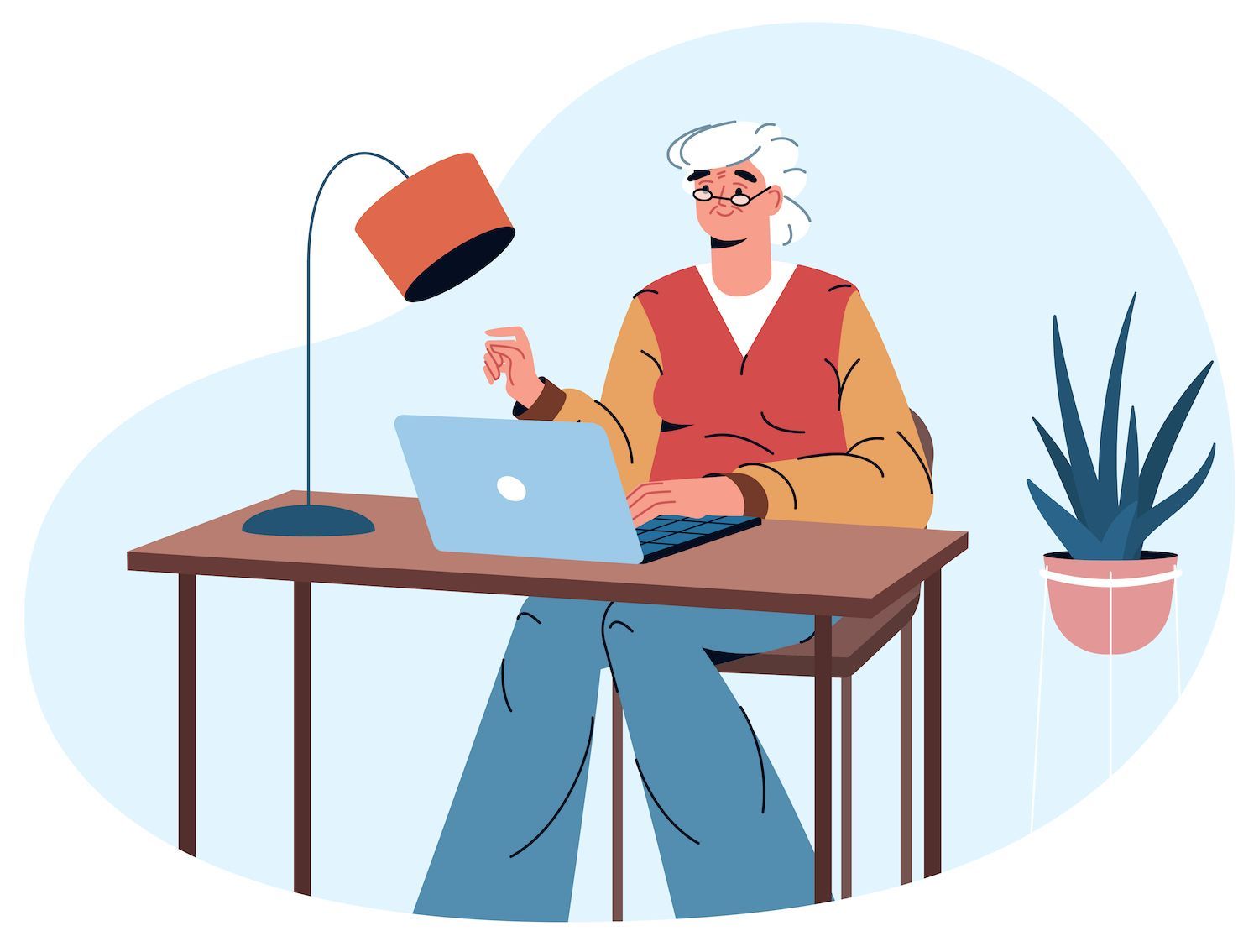
To reduce load time and improve the overall experience of your customers, use a technique referred to by the term lazy load. It's an native Angular feature that allows you to only load the essential components of your website application first and then load additional modules as needed.
In this post, we'll talk about lazy loading and the ways it can increase the speed of your site application.
What exactly do you mean by Lazy Loading?
Lazy loading is a common method of loading for image and video files when websites host a variety of media. Instead of loading all media at once, which can consume a significant amount of bandwidth and bog down the page's load time, these elements are loaded as soon as their location on the webpage is set to be scrolled into view.
Angular is a single-page application framework that is based on JavaScript for a large portion of its capabilities. The library that your application uses of JavaScript can easily become large when the application grows which can lead to a increasing data consumption and load time. In order to speed up the process using lazy loading. This allows you to start by loading the necessary modules, and then delay the loading of the other modules until the time they're required.
The benefits from Lazy Loading in Angular
Lazy loading has advantages to enhance the user experience on your site. These include:
- Faster load time: JavaScript contains instructions to display your webpage and processing the information. Due to this, it's an rendering resource that blocks the rendering. The browser has to wait to load all of JavaScript prior to rendering your webpage. When lazy loading in Angular, the JavaScript is divided into pieces which are loaded in a separate. The chunk that is loaded first contains the logic needed to create the primary portion that makes up the webpage. The page is loaded fast before the rest of the modules load slowly. When you decrease the size of your initial chunk, the site will is faster to load and render.
- Utilize less data by dividing the information into smaller pieces and then processing it in accordance with the requirements it is possible to reduce the bandwidth that you consume.
- Conserved browser resources Because the browser loads only the chunks that are necessary, it will not eat the CPU and memory trying to interpret and render codes that aren't necessary.
Implementing Lazy Loading in Angular
In order to follow the guide, you'll need to have the following things:
- NodeJS installed
- Basic understanding of Angular
Step Up Your Project
NPM install the following command: -g @angular/cli
Then, you can create an application dubbed Lazy loader demo. This is how it works:
HTML0 new demo of lazy loading -- routing
This command will start a brand new Angular project with routing. You'll be working exclusively within the the src/app
folder. This directory contains the source code for the app. This folder contains your main routing file, app-routing.module.ts
. The structure of the folder will be like this:
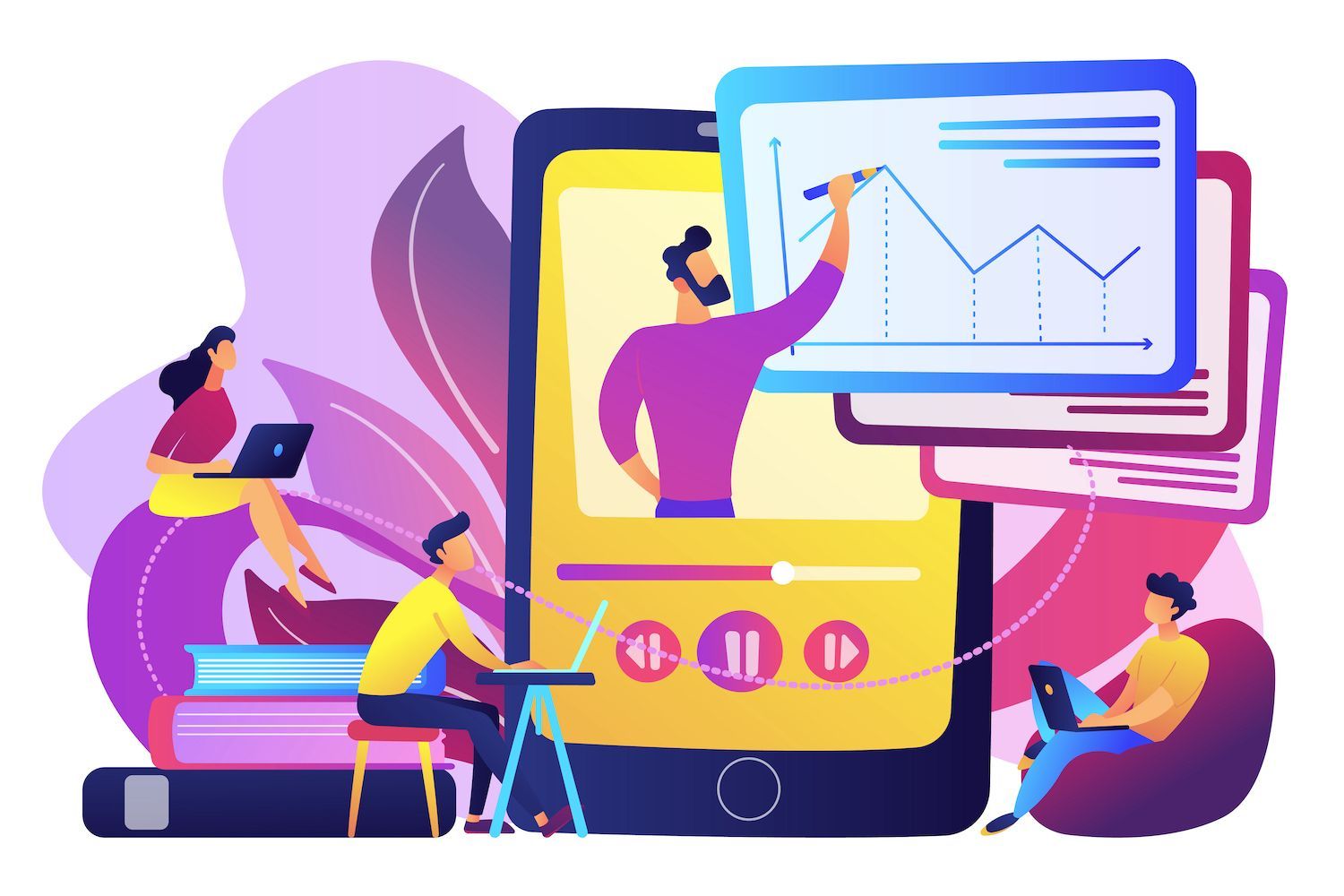
Make a Feature Module using Routes
The next step is to create a feature module that will load slowly. To create the module, you need to run this command:
ng generate module blog --route blog --module app.module
The command will create the new module BlogModule
and will also create a routing. If you open src/app/app-routing.module.ts
, you will see it now looks like this:
import NgModule from '@angular/core'; import RouterModule, Routes from '@angular/router'; const routes: Routes = [ path: 'blog', loadChildren: () => import('./blog/blog.module').then(m => m.BlogModule) ]; @NgModule( imports: [RouterModule.forRoot(routes)], exports: [RouterModule] ) export class AppRoutingModule
The most important part in lazy loading is the third line:
const routes: Routes = [ path: 'blog', loadChildren: () => import('./blog/blog.module').then(m => m.BlogModule) ];
That line is what determines the path. The blog's route makes use of an argument known as"the loadChildren
argument instead of the element
. The loadChildren
argument instructs Angular to make use of lazy loading of the route that is, to start loading the modules dynamically only once the route has been reached, then bring it back to the route. It is identified as a child route, like blog /**. blog/**
is the name of the routing.module.ts
file. Your blog module that you made looks like this:
import NgModule from '@angular/core'; import RouterModule, Routes from '@angular/router'; import BlogComponent from './blog.component'; const routes: Routes = [ path: '', component: BlogComponent ]; @NgModule( imports: [RouterModule.forChild(routes)], exports: [RouterModule] ) export class BlogRoutingModule
The routing file is one route ''
. It resolves to blogs
and redirects towards the BlogComponent. You can add more components, and specify the routes within the file.
For example, if you want to include the ability to gather data from a certain blog, you can make the component using this command:
ng generate component blog/detail
That generates the component that contains the blog's information which is later added to the module for blogs. If you'd like to create an option for it, you simply need to include it in the array of routes:
const routes: Routes = [ path: '', component: BlogComponent , path:"/:title",component: DetailComponent];
This adds a route that resolves for blog/:title
(for example, blog/angular-tutorial
). The list of routes in the bundle are lazy loaded and does not form included in the bundle that was first developed.
Verify Lazy Loading
You can check whether lazy loading is working via running an ng server
by observing the output. In the lower portion of the output, you'll see these results:
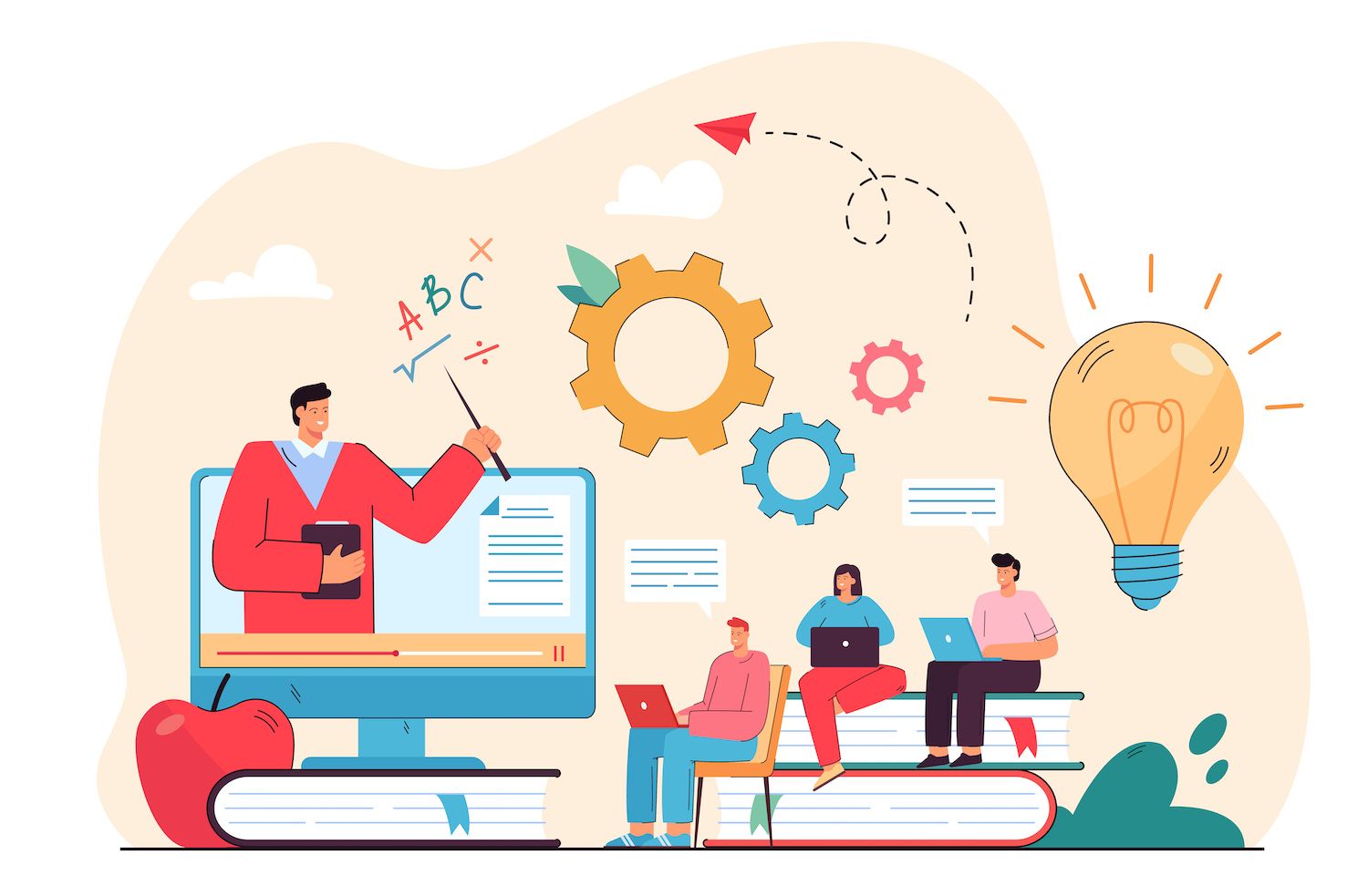
service ng
.The information above is broken down in two sections: Initial Chunk Files
are the ones that load when the site starts loading. Lazy Chunk Files
are lazy loaded. The blog module is explained in the next example.
Verifying for lazy loading through Browser Network Logs
Another way to confirm lazy loading is by using an option known as the Network tab of Firefox's Developer Tools panel. (On Windows, that's the key combination F12 in Chrome and Microsoft Edge, and Ctrlor ShiftI I in Firefox. If you're running the Mac the command is CommandOptionI I. OptionI I in Chrome, Firefox and Safari.)
Select the filter Select the JS
filter, so you only view JavaScript documents that have been uploaded to the web. After the initial load of the app, you should get something like this:
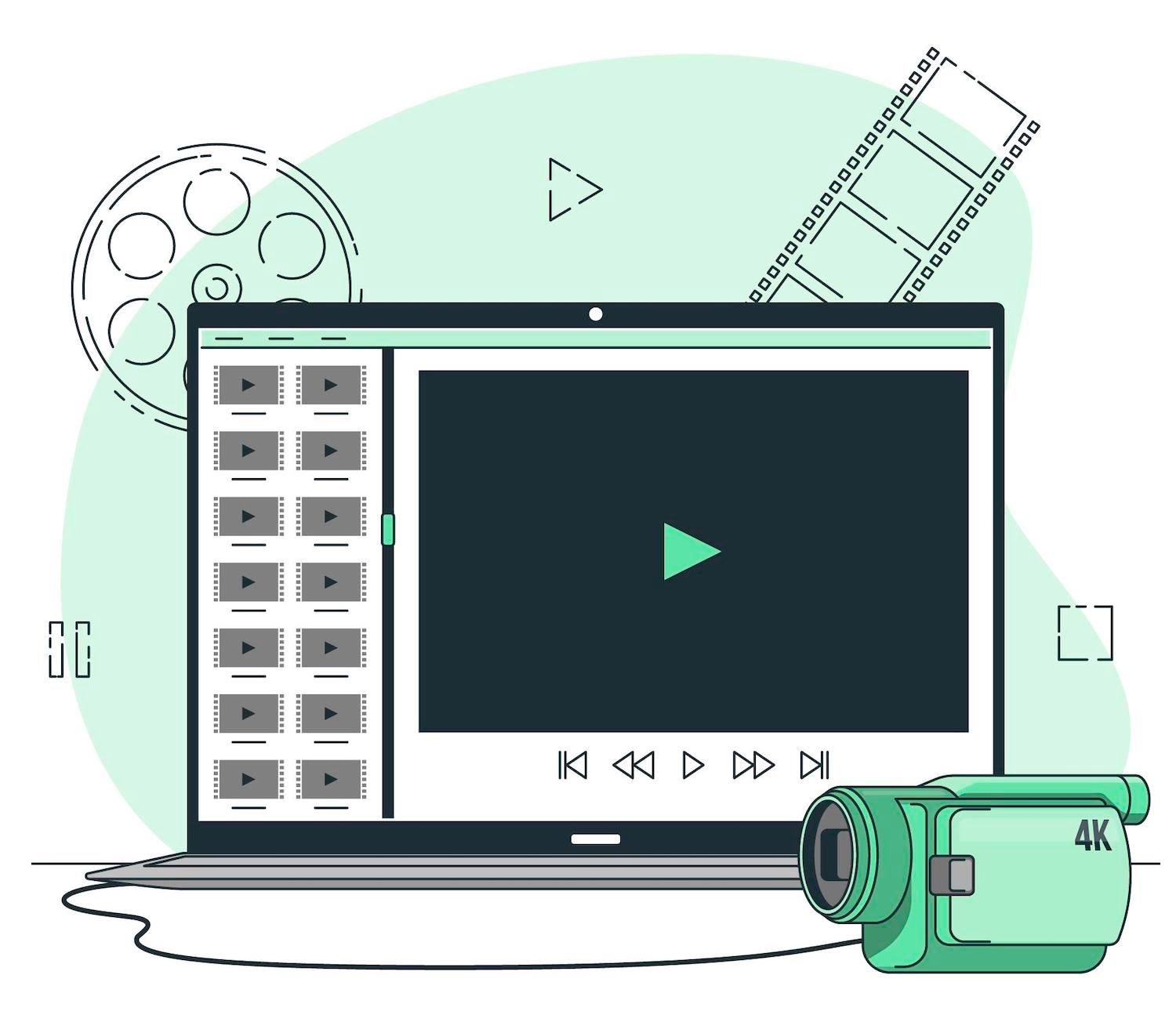
When you navigate to /blog
, you will notice a new chunk, src_app_blog_blog_module_ts.js
, is loaded. This means your module was only requested when you clicked that link, and it is loaded slowly. The network log should appear in the following format:
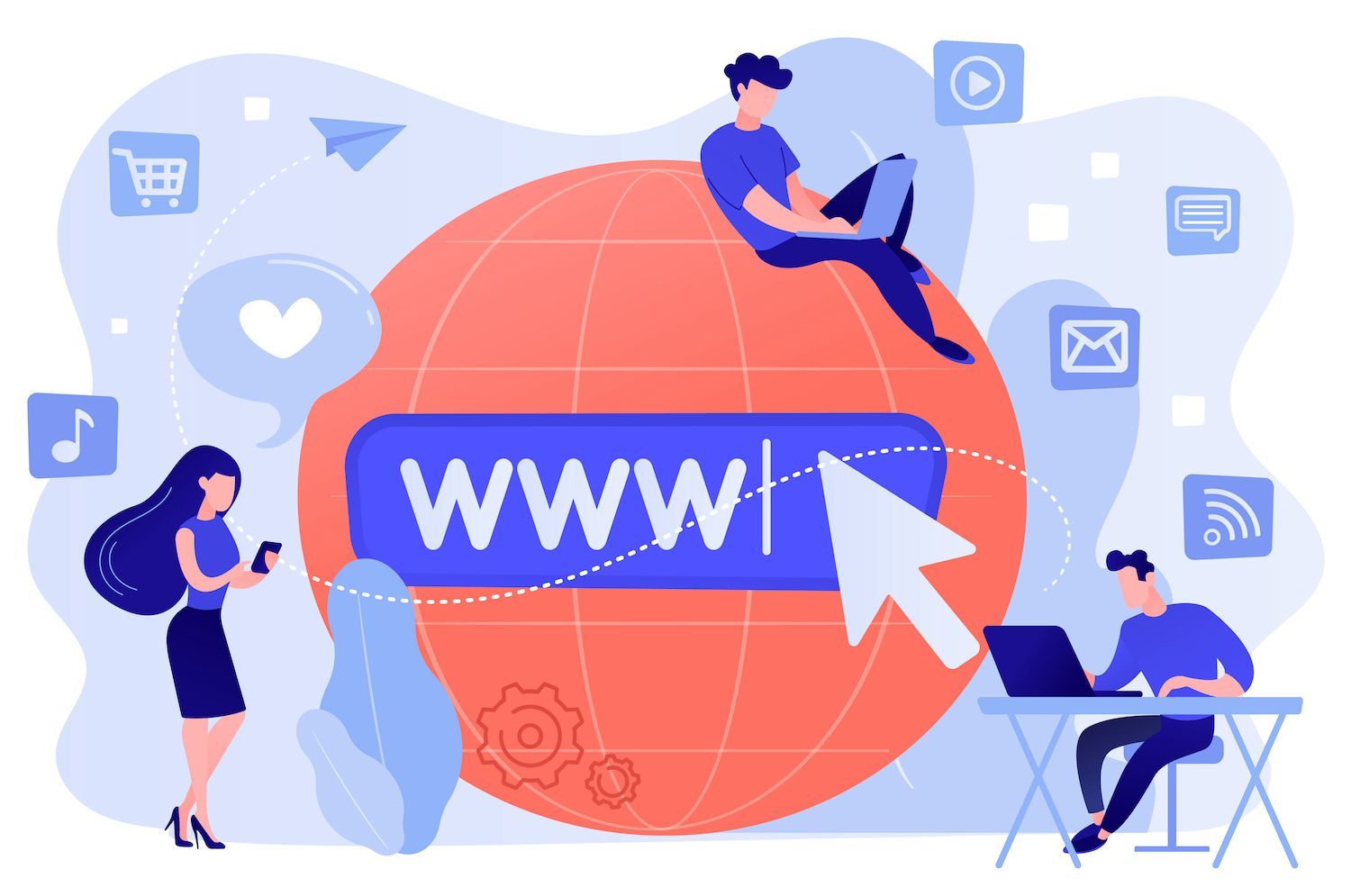
Lazy Loading Vs Eager Loading
To compare the results the performance of a newly loaded module, and then observe how it affects its size, as well as load time. To demonstrate this, you'll create a module that authenticates. It will need a rapid loading time, since authenticating is something that you may need all users to complete.
Make an AuthModule using this command from the CLI
ng generate module auth --routing --module app.module
This generates the module as well as that creates the routing files. The module is also added into the app.module.ts. app.module.ts
file. But, unlike the command we employed to create an application last time, the one we're using now isn't loaded with lazy routes. The module makes use of using the "-routing"
parameter instead of the name of the parameter --route>
. This adds the authentication module to the imports
array of app.module.ts
:
@NgModule( declarations [AppComponent ], imports: [ BrowserModule, AppRoutingModule, AuthModule [added auth module] providers: [, bootstrap: [AppComponent] )
By adding AuthModule to the AppModule imports array indicates that this module has been included in the first chunk files, and is included in the JavaScript bundle. If you want to test the confirmation of your request to be sure, you run ng serve
once more and check the results:
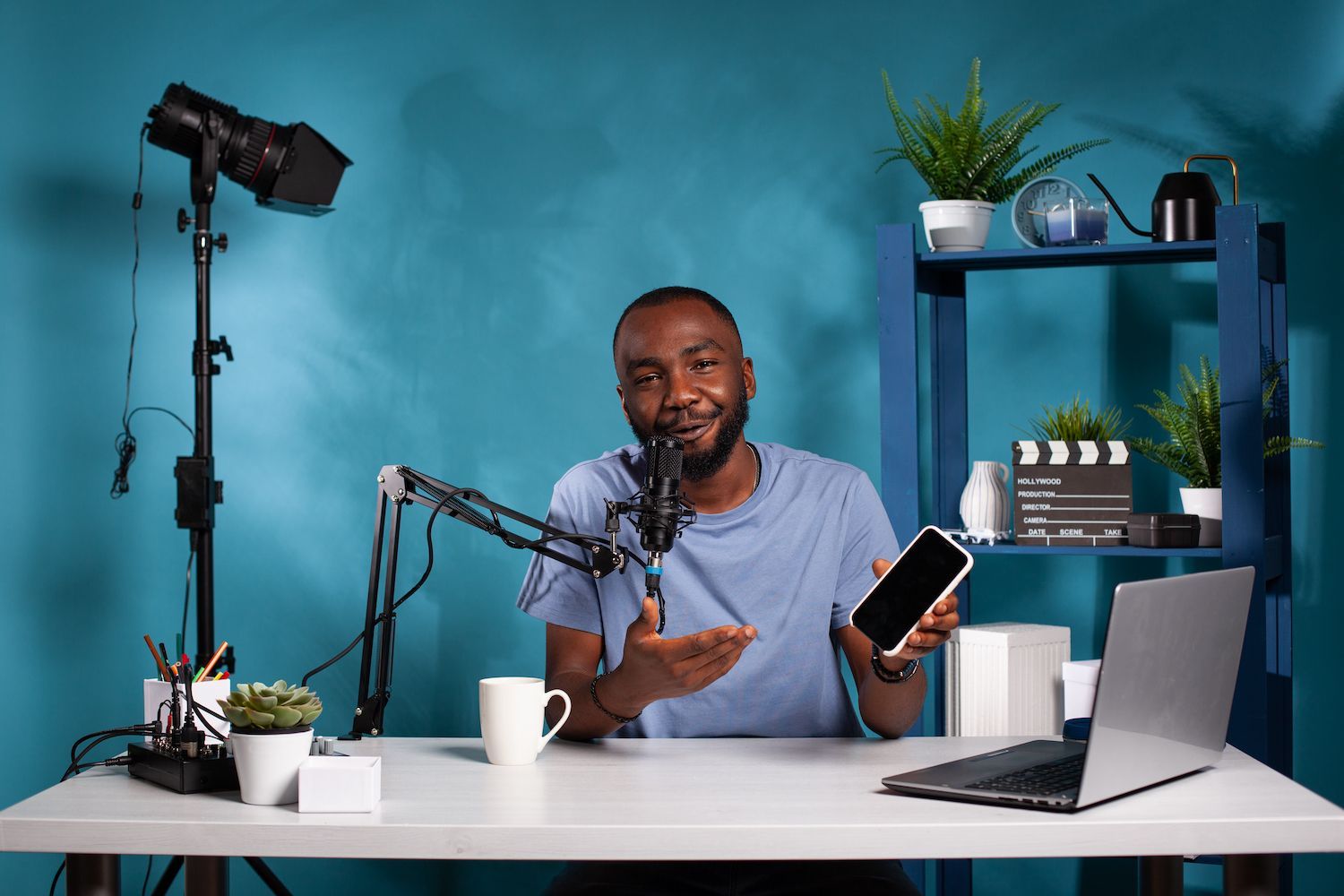
ng serve
command after authentication module is installed.You can observe that the authentication module is not embedded in the lazy chunk files. Additionally, the size of the first bundle is growing. The main.js
file almost has doubled in size. It's increased from 8KB to 1 KB. For this instance, the increase isn't too significant as these components do not contain a lot of code. But, as you fill the components with logic the size of the files will grow and provide a convincing argument to use lazy loading.
Summary
- Simple management and setup on My Dashboard. My dashboard
- Expert assistance 24 hours a day
- The most powerful Google Cloud Platform hardware and network is powered by Kubernetes to guarantee maximum capacity
- Enterprise-level Cloudflare integration for speed as well as security
- The global audience is reached by as many as 35 data centers, as plus 275+ POPs all over the world
This post was first seen on here