Implement Best Practices for a better Game. your game by the 9th of December 2022.
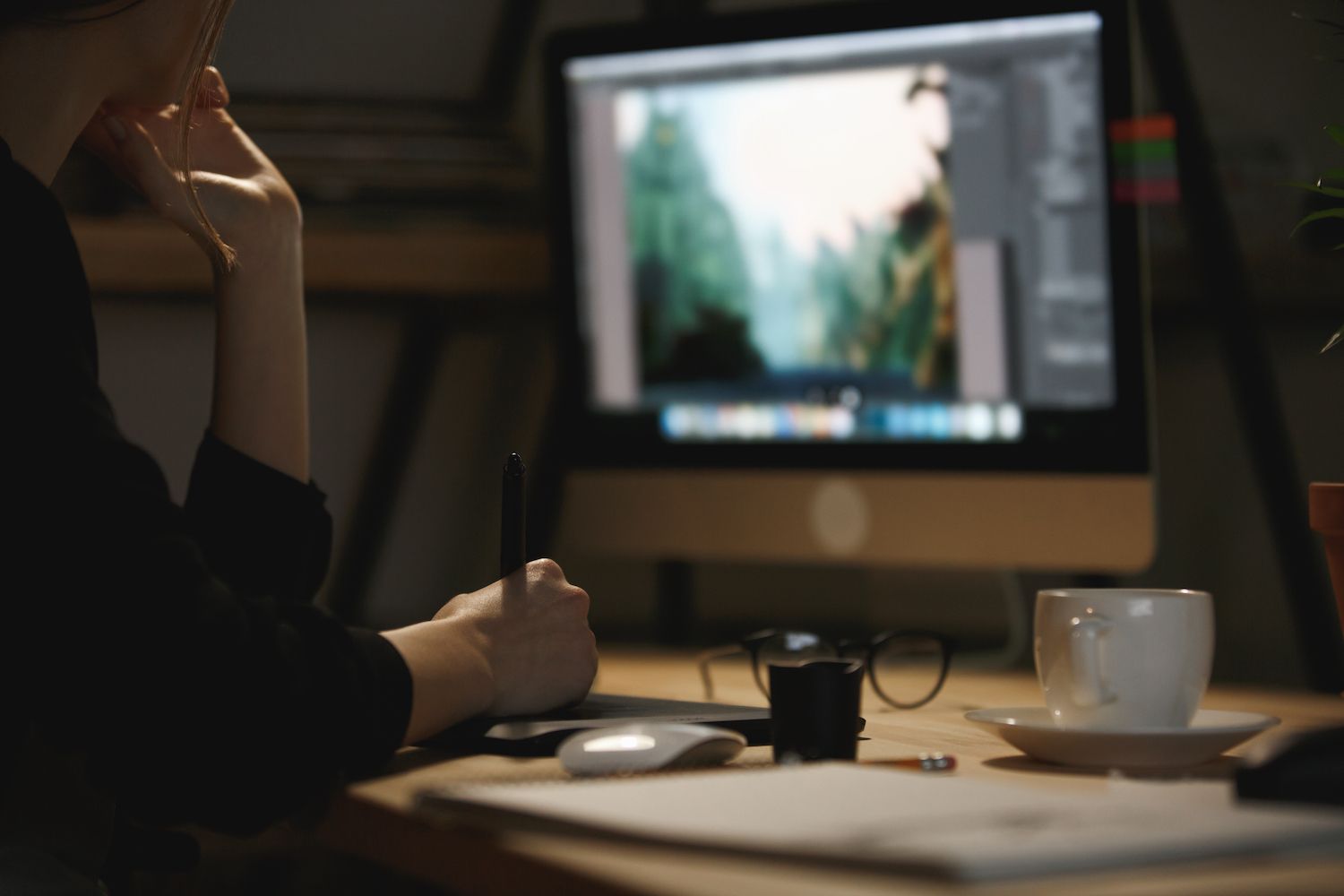
For an React developer, learning how React works isn't the only aspect you need to complete in order to build projects that are easy to use and easy to scale and manageable.
Additionally, you must know about certain conventions to enable you to write concise React code. This will allow you to assist your customers and help you and other developers working for the project to manage the base of code.
In the tutorial video in this tutorial, we'll begin by discussing some typical challenges React developers face. After that, we'll discuss several of the more successful methods to write React codes in a much better way.
Let's get started!
Challenges React Developers Face
You'll be confronted with a variety of challenges in this article can be prevented by following some guidelines, which we'll discuss more in depth later.
We'll start by discussing one of the fundamental problems that plagues newbies.
Conditions To React
There are, in particular, the essential JavaScript concepts and functions to know before getting into React. A few of them, most of which belong to ES6 are:
- Arrow serves a purpose
- Rest operator
- Spread operator
- Modules
- Destructuring
- Methods to array
- Template literals
- Promises
Let
along withconst
variables
The JavaScript topics listed above will provide you with the fundamentals of how React operates.
Furthermore, you'll get to know about the most recent concepts within React like:
- Components
- JSX
- State management
- Props
- Rendering elements
- Event handling
- Conditional rendering
- Lists and keys
- Formulas and validation for forms
- Hooks
- Styling
An understanding of React concepts and the requirements to use the library could enable you to use its capabilities efficiently.
State Management
In JavaScript altering a variable could be as easy as assigning it a completely new value with the operator equal ( =
). Here's an illustration
var x = 300; function updateX() x = 100; updateX(); console.log(x); // 100
In the example code you can see that we have created the variable x
which has a beginning value within the range of 300
.
Employing the equal operator we determined 100 to it. 100
to it. The code was written using an updateX
function.
In React it's possible to modify the value of variables is performed in a different way. Here's how:
load useState from'react'; function App() const [x setX, setX] = useState(300) allow updateX =()=> setX(100) and return ( x Update X) (x Update X) SetX, setX,) SetX, x) SetX setX; export the default App
For updating the status of a variable within React, use the useState
Hook. Three points are important to take note of while using this Hook
- The variable's name
- The function allows you to modify the variable
- The initial value/state that the variable was in at the time of its creation.
In our our example,"x" is the title to the variable. SetX functions that updates the value of x . The contrast to the value (300) for x. x. It is set as a parameter of the function useState:
const [x , setX, setX, x[x]. useState(300)
To update the state of the x
to reflect the present state of x, we utilized the function setX:
function:
import useState from'react' Let updateX =()=> (100);
So, the program updateX
function invokes setX function. setX
function. This function can then be used to set the value for x
to the value of 100.
.
While this technique appears to be a perfect choice for making changes to the state of your variables however, it can add to the quantity of code needed for large projects. The majority of State Hooks make the code hard to track and to comprehend, especially in the case of projects that grow.
Another issue that comes with this State Hook is that these variables created are not used across the various components of the app. It's still necessary to have Props for transferring the information between variables.
Luckily for us, there are libraries designed to manage state management in a way that works with React. You can even define a variable one time and use it anywhere you'd like inside your React application. The libraries include Redux, Recoil, and Zustand.
The challenge with using third-party state management software is that that you'll have to learn the concepts that are different from what you've already learned in React. Redux is a good illustration. It is well-known because of its extensive boilerplate code that caused it to be difficult for newcomers to understand (although it has been streamlined with the Redux Toolkit, which permits you to write fewer codes than with Redux).
Maintainability and Scalability
If the requirements of customers change for the product There is always a need to make changes to the software which makes up the product.
It's sometimes difficult to expand the code you write if it doesn't make it easy for teams to keep up. These issues arise because of using bad practice when writing your program. These practices may seem to work perfectly at first giving you the desired result, but all that's working "for right now" is inefficient for the future and development of your program.
Responding to the most effective methods
In this part we'll review some of the top practices to follow when writing code for React code. Let's get started.
1. Make sure that the Folder Structure is clear
Folder structures help the designers and the users to comprehend the organization of files and other assets used in projects.
Once you've established a good arrangement of your folders, it's easier to locate your way to the right place, saving time as well as helping to avoid confusion. The structure of your folders is different for the preferences of each team, however below are some of the popular folders in React.
Sorting Folders by Features, or Routes
Separating your files into their route and capabilities allows you to organize everything related to a particular feature in the same location. If, for example, there's a dashboard you are using for your users, you can have the JavaScript, CSS, and tests files that are related to the dashboard all in one folder.
Here's an example to demonstrate that:
dashboard/ index.js dashboard.css dashboard.test.js home/ index.js Home.css HomeAPI.js Home.test.js blog/ index.js Blog.css Blog.test.js
It is evident that the core features of the app has all its files and assets saved in the same place.
Similar files can be grouped
Additionally, you can group similar files in the similar folder. It is also possible to create separate folders in order to keep Hooks components, Hooks, as well as the other components. Read this case study
hooks/ useFetchData.js usePostData.js components/ Dashboard.js Dashboard.css Home.js Home.css Blog.js Blog.css
It is not necessary to adhere to these structures in code. If you've got a particular way to order your files, go with the method you prefer. So long as the developers are aware of the understanding of format of the files, you're all set to begin!
2. Create a Structured Import order
If you're React application keeps growing it's inevitable that you'll need to create extra imports. The format of your imports go a long way in helping you understand what constitutes your component.
The common method is to group similar programs with similar programs. This is generally an excellent idea. It is feasible to distinguish external or third-party imports from local imports.
See this illustration:
import Routes, Routes imported from "react-router-dom" toimport the createSlice from "@reduxjs/toolkit" and import the Menu from "@headlessui/react" Inject the Home folder into "./Home" andimport logos from "./logo.svg" Import "./App.css";
In the above example code in the example code above, we have first put third party libraries (these were libraries that we needed to install prior).
Then, we imported the files we had developed locally, like images, stylesheets, and components.
In order to ensure easy comprehension and easy use, the example we've chosen to show doesn't have an enormous codebase, however using this method of imports will help the other developers to understand the design that is your React application better.
It is possible to further organize your local files according to the kinds of files you have in the event that you would like to do -- in other words you can group images, components styles, hooks, and more, in separate areas within your local imports.
The following is an example:
home imports from "./Home" and import "about" in "./About" to import contact from "./Contact" Import the logo image file in "./logo.svg" andimport closeBtn in "./close-btn.svg" to load "./App.css" toimport "Home.css"
3. Name Conventions. Conventions
Naming conventions are a way to increase the readability of code. This is not limited to components' names, but also to your variable names, through to your Hooks.
The React documentation is not an official format for the names of your components. The most commonly utilized naming conventions can be found inside camelCase as well as PascalCase.
PascalCase is used to describe name of component:
import React from 'react Function StudentsList() return ( studentList ) export default StudentList
The above component is called StudentList
, which is much easier to read than StudentList
and Studentlist
.
Want to know the ways we have increased the number of visitors to our website by 1000%?
Join the 20,000+ who receive our newsletter every week. We'll give you insider WordPress techniques!
In contrast on the other hand this camelCase names convention is primarily used to identify hooks, variables, functions as well as arrays etc.
const [firstName, setFirstName] = useState("Ihechikara"); const studentList = []; const studentObject = ; const getStudent = () =>
4. Utilize the Linter
The program analyzes the code you have written and alerts you the rule that has been violated. A word or phrase that is in violation of the rule will usually be highlighted red.
Because every developer is distinct in the way they code, linter tools will help to make sure that codes are homogenous.
Tools for Linter can help to solve bugs swiftly. You can spot spelling mistakes as well as variables declared , yet never used, and additional functions like this. Certain bugs could be solved automatically by modifying the program.
5. Employ Snippet Libraries
Snippet libraries assist developers in developing faster by offering prebuilt code which developers can use frequently.
A good example is the ES7+ React/Redux/React-Native snippets extension, which has a lot of helpful commands for generating prebuilt code. For instance, the case of, for instance, wanting to create an React functional component, without having to type out the entire code, all you have to input is "rfce"
and enter. Enter.
The command above will go further to create a functioning component that has an alias which matches the file's name. We generated the code below using the ES7+ React/Redux/React-Native snippets extension:
import React by calling'react studentList method() return ( studentList ) export default StudentList
Another option for small snippets code that can be useful can be useful is an extension called the Tailwind CSS IntelliSense extension, which helps in creating web pages that are styled with Tailwind CSS. This extension will assist you in autocompletion, by suggesting useful classes, such as syntax highlight and functions to lint. This extension allows you to see what the colors appear as you write.
6. Combining CSS with JavaScript JavaScript
When working on large project, using separate styles of stylesheet files to distinct components may make the organization of files difficult to for users to navigate.
One solution is to blend your CSS with JSX code. You can use frameworks, libraries, or frameworks like Tailwind CSS and Emotion for this.
This is how the styling with Tailwind CSS appears:
edge of resource
The following code gives the element of paragraph a bold font and adds some margin to the right. This is possible this by using the utility classes of the framework.
Here's how you'd design an element with Emotion:
Hello World!
7. Limit Component Creation
One of the main characteristics of React is the code reusability. It's possible to build components and then use the logic for as many times as you want without having to modify the logic.
Keep this in mind while creating your files it is important to limit the number of components you build. Doing so will create a bloated structures of your files with redundant kinds of files that don't need to be there in any way.
Let's use an extremely simple illustration to demonstrate this:
Function UserInfo() return ( My name is Ihechikara. ); export the default UserInfo
The above image shows the user's name. If we could only create one file per user, it would result in an an unreasonable number of documents. (Of course the fact that data about users is used helps make the process easier. In real-world situations, it could be necessary to work with another type of thinking.)
To make our part usable, we could use props. Here's how:
Function UserInfo(UserName) *Export default UserInfo
Once we have that done, we may then use this part and import it for as many times as we'd like.
transfer UserInfo to "./UserInfo" Function App() return ( ); export default app;
Three distinct examples of the UserInfo
component that derives from the code that is created in one file, instead of having distinct file for each user.
8. Implement Lazy Loading
By default, React packs and deploys the entire application. We can alter this behavior with lazy loading or code splitting.
You are able to limit the content of your application that will be loaded at a specific date. It is done by breaking your bundles , and then loading content that is pertinent to the requirements of the users. In this case, for example, you can initially load just the necessary process to allow users to sign-up and then load the necessary logic to the dashboard after users have successfully logged into the system.
9. Utilize Reusable Hooks
Hooks included in React let you harness the additional capabilities of React like interacting with your component's state and running effects in relation to changes in the state of the component. This is possible without having to write class components.
Additionally, we can make Hooks reusable so that they don't require us to write the logic each time that they are used in. This is to create distinct Hooks that are able to be imported anytime within the application.
In the example below, in the next example We'll create a Hook for fetching information from APIs which are not internal:
import useState and useEffect in "react";function useFetchData(url) Const [data setDatadata SetData useState(null) setData useState, setData useState (null)(() = useEffect (()) =fetch(url) .then((res) => res.json()) .then((data) = setData(data)) .catch((err) > console.log(`Error in $err `)); [url]) Return data ; export the default value of useFetchData
The Hook was created that can retrieve data from the APIs mentioned in the previous paragraphs. This hook could be transferred to any part that is part of your system. This eliminates the need to write all that process in each component that requires external data.
The variety of customized Hooks that you are able to create in React is endless, therefore you can choose the best way to use them. Take note that when designing a feature that needs been designed to work across different elements, you should make sure that the functionality is reuseable.
10. Track and Control Errors
There are different ways of handling errors in React like using error boundaries, try and catch blocks or using external libraries like react-error-boundary
.
The built in error boundaries which were introduced in React 16 was a functionality intended specifically for classes, therefore we will not discuss it since it's recommended to use functional components in instead of class components.
However the use of the attempt
or catch
block is only suitable to execute imperative code but it's not a declaration procedure. This means that it's not an option in conjunction with JSX.
Our best recommendation would be to use a library like react-error-boundary. The library offers functions that are wrapped around your component, which can help you detect problems while your React application is being rendered.
11. Examine and verify Your Code
While many might argue that testing doesn't matter when creating an application for the internet, it comes with innumerable advantages. These are just a few examples:
- Finding bugs results in improved code quality.
- Unit tests can be documented to collect data and later references.
- An easy fix for bugs could save users from having to pay developers to eliminate the fire which the flaw could result in if the issue isn't addressed.
12. Utilize Functional Components
The use of functional components using functional components React provides a number of benefits: There is less writing required and it's much easier to read, and it's the current beta version of official React document is currently being refined through the use of functional parts (Hooks) which is why it's a good idea to get used to working with functional components.
Functional components ensure that there is no need to worry regarding how you can use the
or by using classes. You can also keep track of the state of your component by writing less code thanks to Hooks.
A majority of the information that's updated available on React utilizes functional components. This makes it easy to grasp and follow helpful guides and other resources produced by members of the community when there are problems.
13. Be Up-to-Date with Updates to React Version
Over the next few times, new features will be introduced, and old ones will be modified. One of the best ways to track this is to keep an eye on the official documentation.
You can also join React community groups via social networks for information about developments as they occur.
Staying up to date to the most recent version of React can help you identify the best times you should optimize or modify your base code for most efficacious performance.
Additionally, there are other libraries built upon React which you must keep current and using including React Router which can be employed to route data in React. Being aware of the changes these libraries can make will help you make the required modifications to your program as well as make the process easier for everybody working on the project.
In addition, certain functions could be removed and some terms could change as new versions become available. To stay on the safer side, you should always go through the user manual and guides for any modifications.
14. Make use of a fast, secure Hosting Service
If you'd want to make your website application accessible to everyone once you've developed it then you'll have to host it. It's crucial to select an extremely fast and safe hosting service.
Hosting your site gives you access to a variety of tools that can help you control and expand your website easily. The web server where your site's hosted allows that the data locally on your machine to be safely stored on the server. The main benefit hosting your website is that people can see the awesome stuff that you've created.
There's an array of hosting platforms offering no-cost hosting for developers, like Firebase, Vercel, Netlify, GitHub Pages, or other more costly services like Azure, AWS, GoDaddy, Bluehost, and so on.
Summary
While you're developing your next web app with React take these top methods in mind in order to ensure that managing and using the product effortless for both users and your development team.
What other React good practices do have that you don't know about but weren't included in this piece? Comment in the comments below. Have fun coding!
- It's easy to manage and set up My dashboard. My dashboard
- Support is always available.
- The best Google Cloud Platform hardware and network, powered by Kubernetes to provide the highest capacity
- High-end Cloudflare integration to improve speed and security
- Global reach with more than 35 data centers, as well as over 275 POPs around the globe
Article was first seen on here