How to Design A WordPress website using API (r)
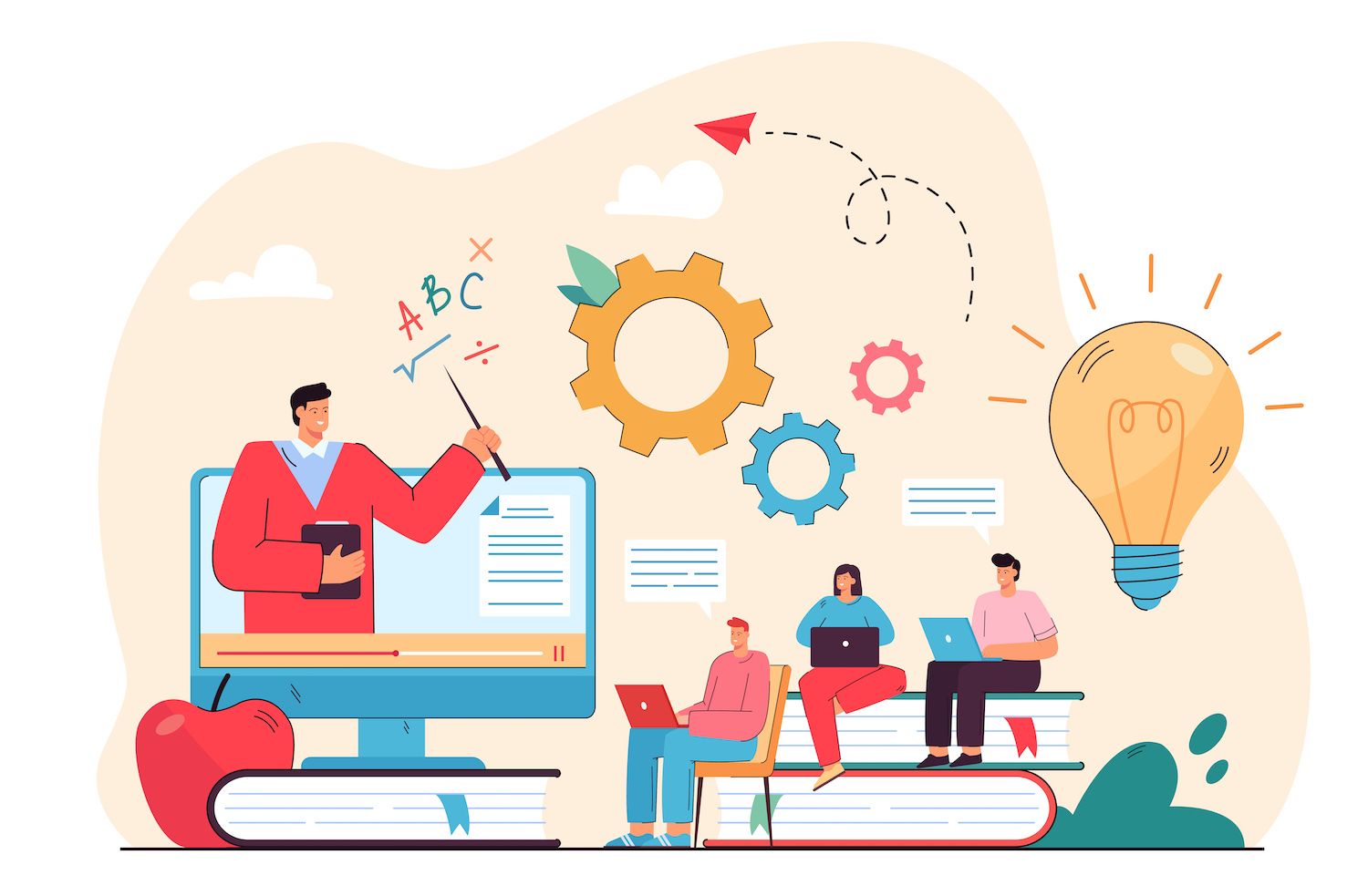
Share on
This is a live demonstration of the web-based site builders application.
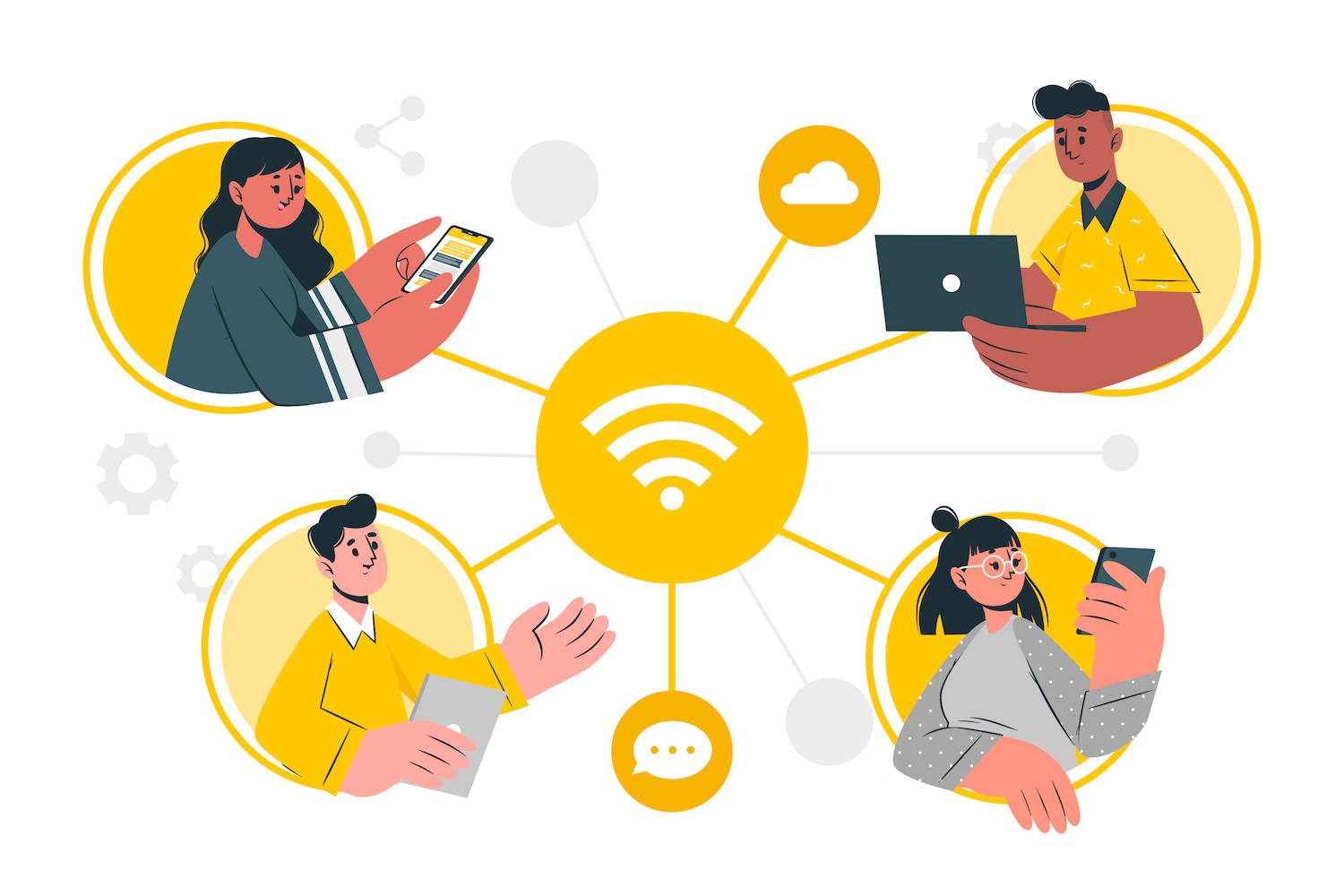
The API: Learning API
To generate an API key. API key
- Log in to Your My Dashboard.
- Check out the API Keys page ( Your name > Corporate settings >>> API Keys).
- Click create API Key.
- Select an expiration date or pick a beginning date as well as the number of hours to allow the key to be used up.
- Make sure the key has a distinct name.
- Click to for Generate.
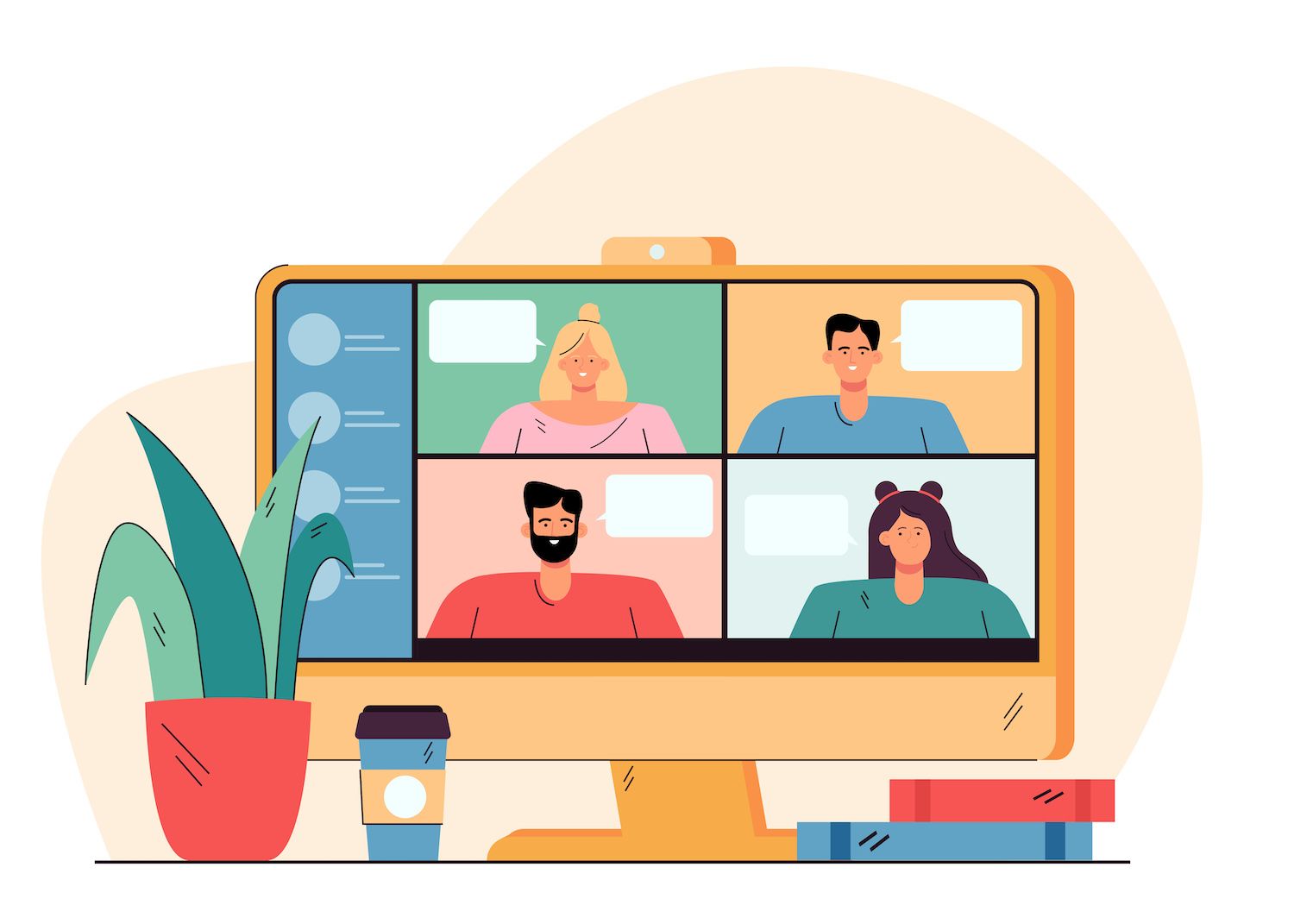
The process of creating a WordPress website by using the API
After you have you've got your API key is in place and you've got your API key, we can create the WordPress site with the API. To do this, make use of site's /sites
endpoint. The payload is expected to be which contains the following details:
- company: It is a parameter that requires a identification number unique for the business that is discovered in My settings. It allows you to determine what the name of the firm connected to WordPress. WordPress website.
- install_mode: This parameter defines the type of WordPress installation. The default setting is "plain," which sets the default WordPress site. You can choose other settings, including "new" for a brand new WordPress installation, and other options dependent on your specific requirements.
- is_subdomain_multisite: This boolean (true/false) parameter specifies whether the WordPress site should be configured as a multisite using subdomains.
- admin_email This parameter is built on the email address of the WordPress administrator. It is used to manage administration tasks, and to receive important emails.
- admin_password This parameter could be utilized to establish passwords for the WordPress administrator user account. Choose a secure password to secure your website.
- admin_user This parameter specifies the username for the WordPress admin account. This is the username used to sign in to the WordPress dashboard as well as to control the site.
- is_multisite: Similar to
is_subdomain_multisite
, this boolean parameter determines whether the WordPress site should be configured as a multisite. - site_title This is a parameter used to determine the name of your WordPress website. It appears at the top of every page of the site. It is possible to change it at a later time.
- woocommerce: This variable indicates whether or not you'd like to enable the WooCommerce plugin when making your WordPress site creation.
- wp_language This parameter is a string value which represents the locale or language of your WordPress site (discover the location of your WordPress locale here).
Once you have a better grasp of the parameters. This is an example of the payload that you provide to the API API will look like:
"company": "54fb80af-576c-4fdc-ba4f-b596c83f15a1", "display_name": "First site", "region": "us-central1", "install_mode": "new", "is_subdomain_multisite": false, "admin_email": "[email protected]", "admin_password": "vJnFnN-~v)PxF[6k", "admin_user": "admin", "is_multisite": false, "site_title": "My First Site", "woocommerce": false, "wordpressseo": false, "wp_language": "en_US"
const createWPSite = async () => const resp = await fetch( `https://api..com/v2/sites`, method: 'POST', headers: 'Content-Type': 'application/json', Authorization: 'Bearer ' , body: JSON.stringify( company: '54fb80af-576c-4fdc-ba4f-b596c83f15a1', display_name: 'First site', region: 'us-central1', install_mode: 'new', is_subdomain_multisite: false, admin_email: '[email protected]', admin_password: 'vJnFnN-~v)PxF[6k', admin_user: 'admin', is_multisite: false, site_title: 'My First Site', woocommerce: false, wordpressseo: false, wp_language: 'en_US' ) ); const data = await resp.json(); console.log(data);
The code is using JavaScript Fetch API. JavaScript Fetch API to send an HTTP POST request to the API for creating an WordPress website. Its CreateWPSite
functionality manages the entire process. In the function, a fetch request is sent to the API's website
endpoint with the information required.
The response is parsed as JSON by using resp.json()
which is logged to the console. Make sure you substitute the YOUR_KEY_HERE value
with your API key. Adjust the payload value as necessary after which you use the CreateWPSite()
to create a WordPress website with the API.
The response looks like:
"operation_id": "sites:add-54fb80af-576c-4fdc-ba4f-b596c83f15a1", "message": "Adding site in progress", "status": 202
Monitoring Operational Activities using the API
When you begin a new site development using the API it's crucial to track the performance of your business. It's possible to do this in a programmatic manner without having to validate the authenticity of your API by using its operation
connection.
For monitoring operations, you can use to monitor operations, use to monitor operations, use the operation_id
that you get at the time of starting an operation, like creating the WordPress website. Send the operation_id
as a parameter the operation's
terminus.
const operationId = 'YOUR_operation_id_PARAMETER'; const resp = await fetch( `https://api..com/v2/operations/$operationId`, method: 'GET', headers: Authorization: 'Bearer ' ); const data = await resp.json(); console.log(data);
If the data response receives via the API The data response is then recorded on the console. The data provided by the response are valuable regarding the status and progress of the task. If, for example, the WordPress website creation process is being completed, the response appears like this:
"status":"status" "message": "Operation in progress", "data": null
Like the method, after the operation is completed successfully, this is the response:
"message": "Successfully finished request", "status": 200, "data": null
The WordPress API allows you to build a WordPress website, and later check its performance through the API. To further enhance it, we could make it more advanced and develop an individual User Interface (UI) capable of managing these processes. So, even those who aren't technical experts can take advantage of the API's capabilities.
Building a React Application To Create an WordPress Website Using API
Prerequisite
Getting Started
For a simpler project set-up process, a starter project is offered for your to use. Start by following these steps to get going:
2. Install the repository on your local computer, and switch it to the starting-files branch with the following command:
Git Checkout starter-files
3. Install the necessary dependencies through this command to install the npm package
. After the installation has been completed, you can launch the project locally on your PC using npm run begin
. This opens the project at http://localhost:3000/.
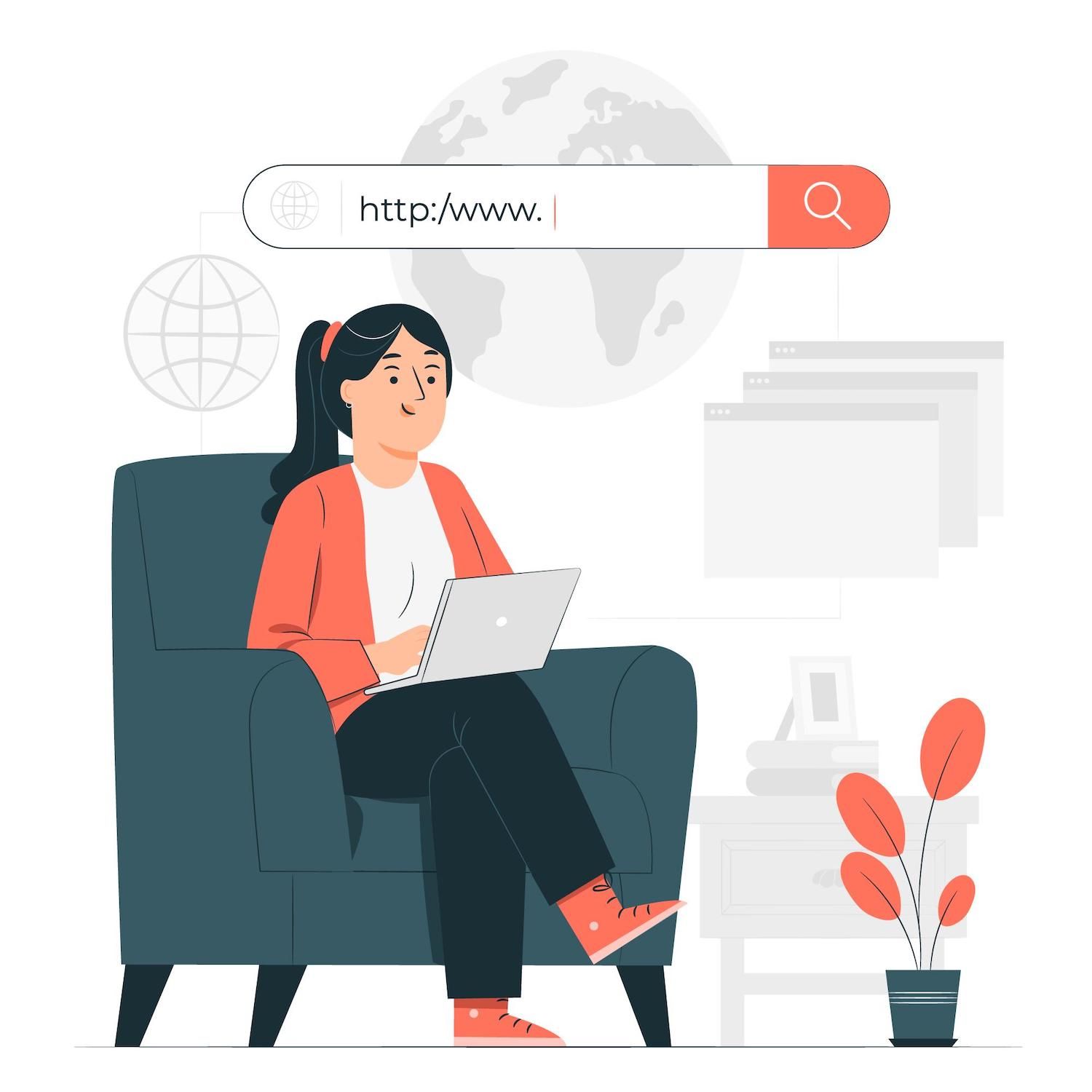
Understanding the Project Files
Within the src directory in this project are two main subfolders: components and Pages. The folder that contains components includes reused components, such as pages, such as those with the header footer and footer which are utilized in both the Homepage as well as the details pages.
This project's primary focus is implementing the logic of the homepage page and the details pages because the design and navigation is already in place.
The Home page has a form that collects information from a variety of fields, which are transmitted to the API. The result of the page is saved in the localStorage (you can explore alternative ways to save the operation ID that is essential to check the status of your operation).
On the Detail page in the Detail page, the ID of operation is pulled from loaclStoage, which is then sent to the API's /operation
endpoint, which is used as an option to check whether the process is at. The application we're using will have a button which allows users to monitor the status on a regular basis (you could use the setInterval
method to automate this process).
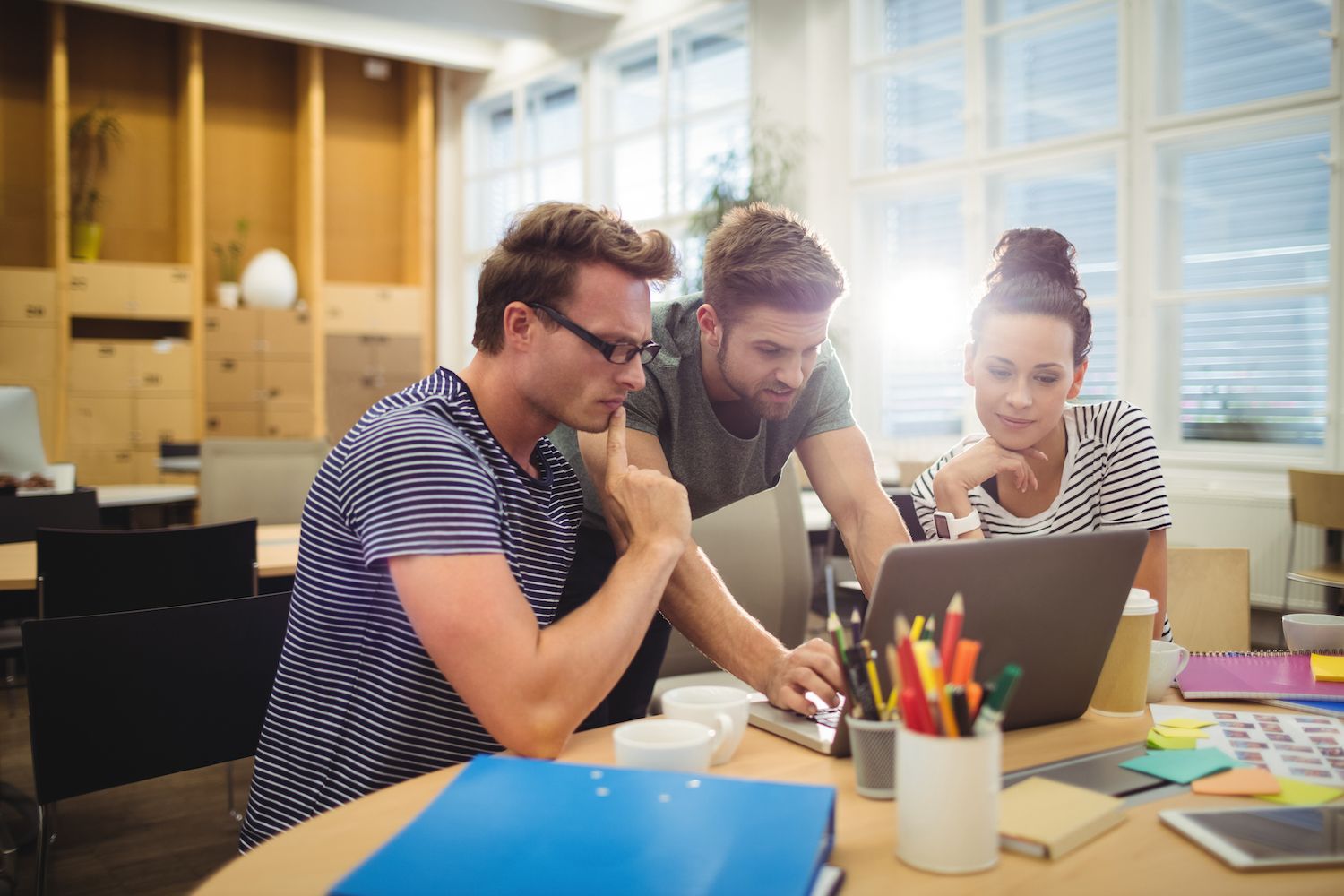
Connecting to the API within React
User interface (UI) is has been put in place, the next step is to process the form submission through your homepage and send a POST request via the API's http://sites
endpoint. The request includes the details of the form in the payload. This allows the development of the WordPress site.
For interaction with the API, you'll need the business ID as well as the API key. After you've obtained the required credentials, try to store them securely as environment variables in the React application.
For setting your environment variables create the .env file inside the root directory of your project. Inside this file, add the following lines:
REACT_APP__COMPANY_ID = 'YOUR_COMPANY_ID' REACT_APP__API_KEY = 'YOUR_API_KEY'
To access these environment variables within your project, you can use the syntax process.env.THE_VARIABLE
. For example, to access the REACT_APP__COMPANY_ID
, you would use process.env.REACT_APP__COMPANY_ID
.
Retrieving Form Data with React
Within Home.jsx Home.jsx file you will find an application form. It is necessary to add an algorithm to the form in order to collect the data, and then verify that it is completed. For retrieving the data from the form in the React application, you could use the controlled component approach and hook. This approach lets you make a state for each form field, and then update it when users enter data.
You must then add the useState
hook that is located on the top of the file.
imports React and useState to "react'
Create an instance of a state variable for each form field within your functional component. As an example, if there is an input field that is meant to be "Site title" Then you can create your state variable siteTitle
:
const [siteTitle] and setSiteTitle] ="useState" ('');
In this instance, the siteTitle
will be the value of a state variable which contains the information in"Site title" input field "Site title" input field. setSiteTitle
is the function that updates state variables.
For the purpose of binding field fields in forms with the appropriate state value, add the value
and add onChange
attributes of each input element. In this case, in"Site title" input field "Site title" input field:
setSiteTitle(event.target.value) />
In this this example it is the values
attribute will be set according to the websiteTitle
state variable. This ensures that your input will display the current value of the title
. When OnChange occurs, the change
event handler set to utilize the setSiteTitle
function. The update of the title of the site
status will show the most recent value every time an user enters data into the input field.
By following this approach for every field of the form you are able to build the needed state variables, and then modify them as users interact through the form. This lets you easily get the data entered once the form has been submitted and then perform any further actions or check the information in the form.
If you do this for each of the fields on your form Your Home.jsx file looks like this:
import Header from '../components/Header'; import Footer from '../components/Footer'; const Home = () => return ( className="title">Site Builder with API className="description"> This is a React app that uses the API to create WordPress sites, without needing to access My dashboard. The form's input is a Form-container input display and the name is used to identify the site. Only used in My and temporary domain className='error-message'>Ensure this has more than 4 characters WordPress site title Appears across the top of every page of the site. It's possible to alter it at a later time. className='error-message'>Ensure this has more than 4 characters WordPress admin username WordPress admin email className='error-message'>Ensure this is a valid email WordPress admin password Ensure you remember this password as it is what you'll use to log into WP-admin Data center location Allows you to place your website in a geographical location closest to your visitors. value=""> /* add options */ Install WooCommerce Install Yoast SEO className='btn'>Create Site ) export default Home; Implementing Form Field Validation Using useRef Hook To enable validation of form fields in React it is possible to take these steps. Focus on creating validation of fields like those in the "Display name" and "WordPress administrator email" fields. Beginning, you need to create references using hooks to regulate how warning message display. Import useRef hook into the UseRef hook and generate references for each field: import useRef' from'react';displayNameRef const = useRef(null) Const wpEmailRef = useRef(null); After that, you attach these refs to element of error messages in the form fields. For example, for"Display Name" for example for the "Display Name" field, you add this ref to the tag span. This is where you'll find the error message Name of display. Helps you to identify the site. Only used in My and temporary domain setDisplayName(e.target.value) /> Ensure this has more than 4 characters The same applies to the "WordPress admin email" field: WordPress admin email setWpAdminEmail(e.target.value) /> className='error-message' ref=wpEmailRef>Ensure this is a valid email Now, we can design validation methods which check input values and decide whether to display the error messages. These are the functions that can be used to use for "Display name" and "WordPress admin email": const checkDisplayName = () => if (displayName.length let regex = /^\w+([.-]?\w+)*@\w+([.-]?\w+)*(\.\w2,3)+$/; if (!wpAdminEmail.match(regex)) wpEmailRef.current.style.display = 'block'; else wpEmailRef.current.style.display = 'none'; The functions are invoked at the time that the relevant input fields change. They match the values of input to validate the values and then update the display of error messages by manipulating the style.display property of the error message's elements. Form for building websites that has been validated. You are free to add additional validations or customize the validation process to suit your needs. Handling Form Submission in React To control the event of submitting a form in the process of creating the website, we will need to accomplish a number of things. In the first place, we add an onSubmit event handler to our form element. Inside the createSite function, we prevent the default form submission behavior by calling event.preventDefault(). This lets us manage the process of submitting forms in a programmatic manner. In order to ensure that the information on the form is correct prior to sending the process of submission it is necessary to validate the techniques CheckDisplayName along with checkWpAdminEmail. This method ensures that the fields conform to the criteria specified. CreateSite = (e) = e.preventDefault(); checkDisplayName(); checkWpAdminEmail(); // Additional logic ; If all validations have been completed and that the fields required contain valid data, proceed through clearing localStorage and make sure that there is an uncluttered environment that can be used to save the API response and the display name. After that, you are able to make an API request through the API with the fetch function. The request is a POST method to the https://api..com/v2/sites endpoint. Make sure you include all the relevant headers and payload as JSON. const createSiteWithAPI = async () => const resp = await fetch( `$APIUrl/sites`, method: 'POST', headers: 'Content-Type': 'application/json', Authorization: `Bearer $process.env.REACT_APP__API_KEY` , body: JSON.stringify( company: `$process.env.REACT_APP__COMPANY_ID`, display_name: displayName, region: centerLocation, install_mode: 'new', is_subdomain_multisite: false, admin_email: wpAdminEmail, admin_password: wpAdminPassword, admin_user: wpAdminUsername, is_multisite: false, site_title: siteTitle, woocommerce: false, wordpressseo: false, wp_language: 'en_US' ) ); // Handle the response data ; The payload is comprised of various information fields that the API requires, such as your company's ID, display name, region configuration mode, admin email, admin password etc. The values come from the corresponding state variables. Following the API request we await the response with await resp.json() in order to take the data relevant to the request. We create a new object newData, with the operation ID and display name, which is then stored in the localStorage using localStorage.setItem. const createSiteWithAPI = async () => const resp = await fetch( // Fetch request here ); const data = await resp.json(); let newData = operationId: data.operation_id, display_name: displayName ; localStorage.setItem('state', JSON.stringify(newData)); navigate('/details'); In the end, we use the createSiteWithAPI function to ensure that, when users fill out the form and clicks the button to create a WordPress website is built with the help of API. Additionally, in the code, it's mentioned that users are redirected to the details.jsx page to keep track of what's happening making use of the API. In order for the navigation feature for use it is necessary to install UseNavigate into react-router.dom and then set it up. Note: You can locate the complete source code for this page on the GitHub repository. The implementation of Operation Status Check with the API To determine the condition of the operation by using the API using the operation ID that was recorded in the localStorage. This operation ID is retrieved from the localStorage using JSON.parse(localStorage.getItem('state')) and assigned to a variable. To check the operation status, make another API request to the API by sending a GET request to the /operations/operationId endpoint. This request will include the needed headers such as the Authorization header, which has an API Key. const [operationData, setOperationData] = useState( message: "Operation in progress" ); const APIUrl = 'https://api..com/v2'; const stateData = JSON.parse(localStorage.getItem('state')); const checkOperation = async () => const operationId = stateData.operationId; const resp = await fetch( `$APIUrl/operations/$operationId`, method: 'GET', headers: Authorization: `Bearer $process.env.REACT_APP__API_KEY` ); const data = await resp.json(); setOperationData(data); Once we receive an answer, we extract all relevant information from the response by using await resp.json(). The operation data is then updated in the state using setOperationData(data). In the return statement of the component, we display the operation message using operationData.message. We also provide a button that allows users to create the check of status for the operation by using the operation check. In addition, if the operational status is that it has successfully finished then users are able to make use of the hyperlinks for an access point to WordPress administrator as well as the site's. The links are constructed using the stateData.display_name obtained from localStorage. Open WordPress admin Open WordPress admin URL Opening URL Open WordPress admin URL opening URL Open WordPress admin Open URL Clicking on these links allows you to open the WordPress admin page and site URL and the site URL within a new tab. It allows users to access them directly without having go to My. This allows you to create your own WordPress website easily using an application that is designed specifically for your needs. How Do You Deploy Your React-Based Application In order to enable your repository to follow these steps: Create or log to an account on the My dashboard. In the left-hand sidebar on the right sidebar, click "Applications" and then "Add services". Select "Application" from the dropdown menu to deploy the React application to . The window with a modal menu that opens up select the repository that you want to create. If you've got several branches, you can select which branch you wish to set up and give an application name. Select any of the data center locations from the available 25 choices. The system will automatically detect the start command for your application. Set up environment variables for My before you deploy. After you have initiated the installation process of your app and then you can begin the process of deployment and typically takes about a couple of minutes. A successful deployment generates a link to your application, like https://site-builder-ndg9i..app/. Summary In this video you've learnt how make use of the API in order to develop the foundation of a WordPress website and then incorporate the API into the React application. Always remember to ensure that you secure your API keys in a secure. If you suspect that you've disclosed it to the public, remove the key, and then create another one. What are the endpoints you'd prefer to have included in the API within the next few years? What would you like to see us develop using the API? Tell us via the comments.
This post was posted on here